function [GO, GF] = gabor2(I, gamma, lambda, b, theta, phi, shape);
时间: 2024-05-31 15:09:41 浏览: 11
这是一个 MATLAB 函数,用于生成 Gabor 滤波器。下面是参数的解释:
- I:输入图像
- gamma:Gabor 滤波器的纵横比
- lambda:Gabor 滤波器的波长
- b:Gabor 滤波器的带宽
- theta:Gabor 滤波器的方向(以弧度为单位)
- phi:Gabor 滤波器的相位偏移
- shape:输出滤波器的形状,可以是 'valid'、'same' 或 'full'
函数的输出是两个矩阵:GO 和 GF。GO 是实部,GF 是虚部。可以将它们合并为一个复数矩阵,用于对图像进行 Gabor 滤波。
相关问题
function [G,gabout] = gaborfilter(I,Sx,Sy,f,theta) if nargin < 5 Sx = 3; Sy = 2; f = 16; theta = pi/2; end
这段代码是 Gabor 滤波的实现函数。其中输入参数 I 是待滤波的图像,Sx 和 Sy 是 Gabor 滤波器的尺度参数,f 是频率参数,theta 是方向参数。如果输入参数的个数小于 5,则使用默认参数值进行滤波。接着,判断输入的图像是否为 RGB 彩色图像,如果是,则使用 rgb2gray 函数将其转化为灰度图像。最后,调用 gaborconvolve 函数进行 Gabor 滤波,并返回滤波后的结果 G 和滤波器响应结果 gabout。
[~,T] = gaborfilter(I);
这是一段MATLAB代码,用于对图像I进行Gabor滤波,并返回滤波后的图像和滤波器的参数。具体步骤如下:
1. 调用gaborfilter函数对图像I进行Gabor滤波,该函数返回两个值:滤波后的图像和滤波器的参数。
2. 使用MATLAB中的“~”符号表示忽略第一个返回值,将第二个返回值(即滤波器的参数)赋值给变量T。
该代码通常用于图像处理领域,Gabor滤波器被广泛应用于纹理分析和特征提取等领域。
相关推荐
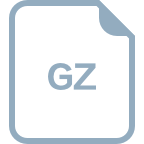
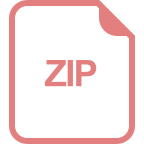
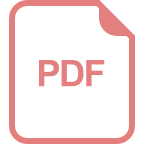













