在c++中用调用函数的反法写一个通讯录
时间: 2024-05-08 10:22:03 浏览: 9
以下是一个简单的通讯录程序示例,使用了函数调用和反射机制:
```c++
#include <iostream>
#include <vector>
#include <string>
#include <map>
using namespace std;
// 定义联系人结构体
struct Contact {
string name;
string phone;
string email;
};
// 定义通讯录类
class AddressBook {
private:
vector<Contact> contacts;
map<string, int> nameToIndex; // 姓名到下标的映射表
public:
// 添加联系人
void addContact(string name, string phone, string email) {
Contact contact = {name, phone, email};
contacts.push_back(contact);
nameToIndex[name] = contacts.size() - 1;
}
// 删除联系人
void deleteContact(string name) {
if (nameToIndex.find(name) != nameToIndex.end()) {
int index = nameToIndex[name];
contacts.erase(contacts.begin() + index);
nameToIndex.erase(name);
// 更新映射表
for (auto it = nameToIndex.begin(); it != nameToIndex.end(); it++) {
if (it->second > index) {
it->second--;
}
}
}
}
// 查找联系人
Contact findContact(string name) {
if (nameToIndex.find(name) != nameToIndex.end()) {
int index = nameToIndex[name];
return contacts[index];
} else {
Contact contact = {"", "", ""};
return contact;
}
}
// 列出所有联系人
void listAllContacts() {
for (int i = 0; i < contacts.size(); i++) {
cout << contacts[i].name << "\t" << contacts[i].phone << "\t" << contacts[i].email << endl;
}
}
// 反射函数,根据函数名调用对应的函数
void callFunc(string funcName, vector<string> args) {
if (funcName == "addContact") {
if (args.size() == 3) {
addContact(args[0], args[1], args[2]);
} else {
cout << "Usage: addContact name phone email" << endl;
}
} else if (funcName == "deleteContact") {
if (args.size() == 1) {
deleteContact(args[0]);
} else {
cout << "Usage: deleteContact name" << endl;
}
} else if (funcName == "findContact") {
if (args.size() == 1) {
Contact contact = findContact(args[0]);
if (contact.name == "") {
cout << "Contact not found" << endl;
} else {
cout << contact.name << "\t" << contact.phone << "\t" << contact.email << endl;
}
} else {
cout << "Usage: findContact name" << endl;
}
} else if (funcName == "listAllContacts") {
if (args.size() == 0) {
listAllContacts();
} else {
cout << "Usage: listAllContacts" << endl;
}
} else {
cout << "Unknown function: " << funcName << endl;
}
}
};
int main() {
AddressBook book;
string line;
while (getline(cin, line)) {
vector<string> tokens;
string token;
for (int i = 0; i < line.length(); i++) {
if (line[i] == ' ') {
tokens.push_back(token);
token.clear();
} else {
token += line[i];
}
}
tokens.push_back(token);
if (tokens.size() == 0) {
continue;
}
string funcName = tokens[0];
tokens.erase(tokens.begin());
book.callFunc(funcName, tokens);
}
return 0;
}
```
这个程序中,我们定义了一个 `AddressBook` 类来管理联系人,包括添加、删除、查找和列出所有联系人等功能。为了实现反射机制,我们定义了一个 `callFunc` 函数,根据函数名调用对应的函数。
在 `main` 函数中,我们通过读取用户输入的方式来调用各个函数。
例如,要添加一个名为 Alice 的联系人,可以在命令行中输入:
```
addContact Alice 123456789 alice@example.com
```
这会调用 `callFunc` 函数,传入函数名 `addContact` 和参数列表 `{"Alice", "123456789", "alice@example.com"}`,然后在 `callFunc` 函数中调用 `addContact` 函数。
需要注意的是,这个程序只是一个简单的示例,没有做任何输入检查和错误处理。实际应用中,需要根据具体需求进行修改和完善。
相关推荐
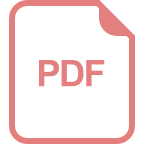
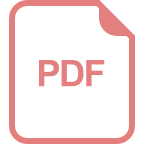
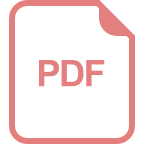














