python如何将文本生成词云图
时间: 2023-11-22 09:04:56 浏览: 100
生成词云图需要用到第三方库 wordcloud,首先需要用 pip 安装该库。安装命令为:
```
pip install wordcloud
```
安装完成后,可以按照以下步骤生成词云图:
1.读取文本文件并处理文本数据:
```
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
text = open('text.txt', encoding='utf-8').read()
text = ' '.join(jieba.cut(text))
```
其中,`jieba.cut`函数用于对文本进行分词处理,返回分词后的结果。
2.生成词云图:
```
wordcloud = WordCloud(font_path='msyh.ttc', background_color='white').generate(text)
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis("off")
plt.show()
```
其中,`font_path`参数用于设置字体文件路径,`background_color`参数用于设置背景颜色,默认为黑色。
执行以上代码后,就可以得到一个简单的词云图了。
相关问题
python将文本生成词云图
生成词云图可以使用Python中的第三方库wordcloud。首先需要安装该库,可以使用以下命令进行安装:
```
pip install wordcloud
```
安装完成后,可以使用以下代码生成词云图:
```python
import jieba
from wordcloud import WordCloud
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 使用结巴分词进行分词
cut_text = jieba.cut(text)
result = ' '.join(cut_text)
# 生成词云图
wc = WordCloud(font_path='simhei.ttf', width=800, height=600, background_color='white')
wc.generate(result)
wc.to_file('wordcloud.png')
```
其中,需要先读取文本文件,然后使用结巴分词库进行分词。最后使用WordCloud库生成词云图,并保存为图片文件。在生成词云图时,可以指定字体、宽度、高度、背景色等属性。
python输入文本生成词云图
好的,生成词云图可以使用 Python 中的 `wordcloud` 库。首先,需要安装 `wordcloud` 库,可以使用以下命令进行安装:
```
pip install wordcloud
```
然后,可以使用以下代码生成词云图:
```python
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 生成词云图
wordcloud = WordCloud(width=800, height=800, background_color='white').generate(text)
# 显示词云图
plt.figure(figsize=(8,8))
plt.imshow(wordcloud)
plt.axis('off')
plt.show()
```
其中,`text.txt` 是要生成词云图的文本文件,需要将其放在与代码文件同一目录下。可以根据需要调整词云图的大小和背景颜色等参数。执行代码后,将会弹出一个窗口显示生成的词云图。
阅读全文
相关推荐
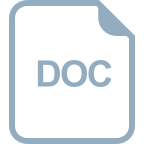
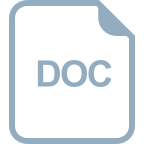
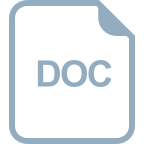
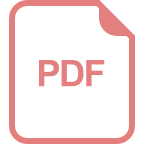


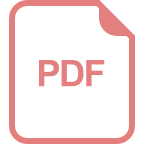
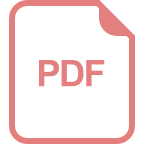








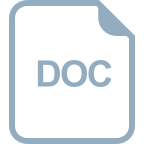