构建一个包含3层全连接层的深度神经网络
时间: 2024-03-02 10:50:28 浏览: 60
好的,下面是构建一个包含3层全连接层的深度神经网络的步骤:
1. 导入所需的库和模块:
```python
import numpy as np
import tensorflow as tf
```
2. 定义输入和输出的维度:
```python
input_dim = 100
hidden1_dim = 50
hidden2_dim = 25
output_dim = 10
```
3. 定义输入占位符:
```python
inputs = tf.placeholder(tf.float32, [None, input_dim])
```
4. 定义第一层全连接层:
```python
# 定义第一层权重和偏置
weights1 = tf.Variable(tf.truncated_normal([input_dim, hidden1_dim], stddev=0.1))
biases1 = tf.Variable(tf.constant(0.1, shape=[hidden1_dim]))
# 计算第一层的输出
hidden1 = tf.nn.relu(tf.matmul(inputs, weights1) + biases1)
```
5. 定义第二层全连接层:
```python
# 定义第二层权重和偏置
weights2 = tf.Variable(tf.truncated_normal([hidden1_dim, hidden2_dim], stddev=0.1))
biases2 = tf.Variable(tf.constant(0.1, shape=[hidden2_dim]))
# 计算第二层的输出
hidden2 = tf.nn.relu(tf.matmul(hidden1, weights2) + biases2)
```
6. 定义输出层全连接层:
```python
# 定义输出层权重和偏置
weights3 = tf.Variable(tf.truncated_normal([hidden2_dim, output_dim], stddev=0.1))
biases3 = tf.Variable(tf.constant(0.1, shape=[output_dim]))
# 计算输出层的输出
outputs = tf.matmul(hidden2, weights3) + biases3
```
7. 定义损失函数和优化器:
```python
# 定义损失函数(使用交叉熵损失函数)和优化器(使用Adam优化器)
labels = tf.placeholder(tf.float32, [None, output_dim])
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(labels=labels, logits=outputs))
optimizer = tf.train.AdamOptimizer(learning_rate=0.001).minimize(cross_entropy)
```
8. 训练模型:
```python
# 加载数据集,然后使用feed_dict将数据传递给模型
# 定义会话
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
# 迭代训练
for i in range(num_iterations):
batch_inputs, batch_labels = get_next_batch(batch_size)
sess.run(optimizer, feed_dict={inputs: batch_inputs, labels: batch_labels})
# 使用测试集评估模型
test_inputs, test_labels = get_test_data()
accuracy = sess.run(accuracy_op, feed_dict={inputs: test_inputs, labels: test_labels})
```
以上就是构建一个包含3层全连接层的深度神经网络的步骤。
阅读全文
相关推荐
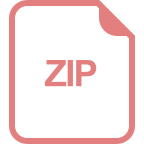
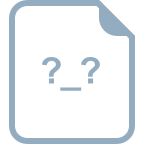
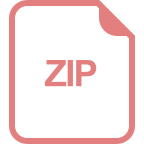
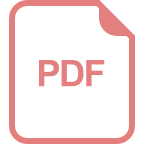
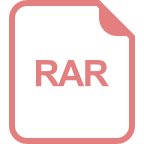
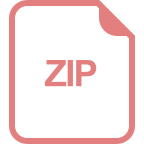
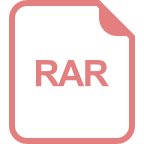
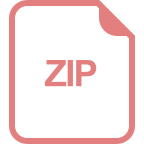
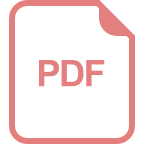
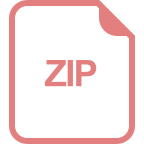
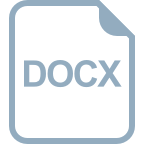
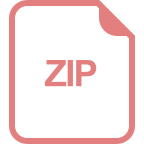
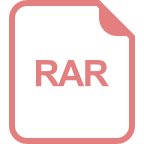
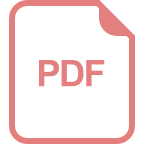
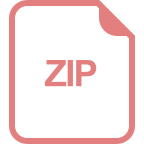
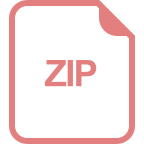
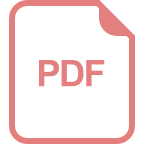
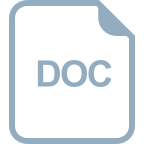