基于python实现与电脑对弈的五子棋游戏。棋盘大小为15*15,双方分别使用黑白两色棋子,下在棋盘竖线与横线的交叉点上。先形成任何方向(横/纵/斜)的5子以上连线者获胜。 用户方通过交互完成与程序的五子棋对弈基本功能: 完成的系统需要实现以下功能: 1用户可以选择先手还是后手: 2胜负状态的判断; 3.有棋子落下后棋盘状态的更新与显示; 4电脑方的下棋位置的决策机制 5.双方平局的判断(无空位落子)。 6.有简易的UI界面 7.电脑方的决策机制可以较为简单 8.给出实现代码
时间: 2024-03-17 20:45:16 浏览: 88
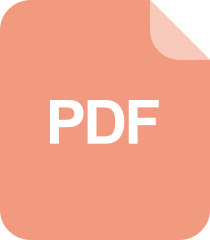
python五子棋游戏的设计与实现

以下是基于Python实现的五子棋游戏代码,包含用户交互、胜负状态判断、棋盘状态更新与显示、电脑下棋决策机制、平局判断和简易UI界面等功能:
```python
import random
import tkinter as tk
class Chessboard:
def __init__(self, master, size=15, width=30, bg='white', fg='black', start='user'):
self.master = master
self.size = size
self.width = width
self.bg = bg
self.fg = fg
self.start = start
self.current_player = start
self.chessboard = [[0] * size for _ in range(size)]
self.create_widgets()
def create_widgets(self):
self.canvas = tk.Canvas(self.master, bg=self.bg, width=self.size * self.width, height=self.size * self.width)
self.canvas.pack(side=tk.LEFT)
for i in range(self.size):
self.canvas.create_line(self.width * i, 0, self.width * i, self.size * self.width)
self.canvas.create_line(0, self.width * i, self.size * self.width, self.width * i)
self.canvas.bind('<Button-1>', self.user_play)
self.message = tk.Label(self.master, text='Black chess plays first', font=('Arial', 12), bg=self.bg, fg=self.fg)
self.message.pack(side=tk.TOP, pady=5)
self.restart_button = tk.Button(self.master, text='Restart', font=('Arial', 12), bg=self.bg, fg=self.fg, command=self.restart)
self.restart_button.pack(side=tk.TOP, pady=5)
def user_play(self, event):
if self.current_player == 'computer':
return
x, y = event.x // self.width, event.y // self.width
if self.chessboard[x][y] != 0:
return
self.chessboard[x][y] = 1 if self.current_player == 'user' else 2
self.draw_piece(x, y)
if self.check_win(x, y):
self.message.config(text=f'{self.current_player.capitalize()} wins!')
self.canvas.unbind('<Button-1>')
elif self.check_draw():
self.message.config(text='Draw!')
self.canvas.unbind('<Button-1>')
else:
self.current_player = 'computer'
self.message.config(text='Computer is thinking...')
self.master.after(1000, self.computer_play)
def computer_play(self):
x, y = self.get_computer_move()
self.chessboard[x][y] = 2 if self.current_player == 'user' else 1
self.draw_piece(x, y)
if self.check_win(x, y):
self.message.config(text=f'{self.current_player.capitalize()} wins!')
self.canvas.unbind('<Button-1>')
elif self.check_draw():
self.message.config(text='Draw!')
self.canvas.unbind('<Button-1>')
else:
self.current_player = 'user'
self.message.config(text='Black chess plays first' if self.current_player == 'user' else 'Computer plays first')
def get_computer_move(self):
# Random strategy
empty_cells = [(i, j) for i in range(self.size) for j in range(self.size) if self.chessboard[i][j] == 0]
x, y = random.choice(empty_cells)
return x, y
def check_win(self, x, y):
directions = [(0, 1), (1, 0), (1, 1), (1, -1)]
for dx, dy in directions:
count = 1
tx, ty = x, y
while tx + dx >= 0 and tx + dx < self.size and ty + dy >= 0 and ty + dy < self.size and self.chessboard[tx+dx][ty+dy] == self.chessboard[x][y]:
count += 1
tx += dx
ty += dy
tx, ty = x, y
while tx - dx >= 0 and tx - dx < self.size and ty - dy >= 0 and ty - dy < self.size and self.chessboard[tx-dx][ty-dy] == self.chessboard[x][y]:
count += 1
tx -= dx
ty -= dy
if count >= 5:
return True
return False
def check_draw(self):
return all(self.chessboard[i][j] != 0 for i in range(self.size) for j in range(self.size))
def draw_piece(self, x, y):
if self.chessboard[x][y] == 1:
self.canvas.create_oval(x * self.width + 2, y * self.width + 2, (x + 1) * self.width - 2, (y + 1) * self.width - 2, fill='black')
elif self.chessboard[x][y] == 2:
self.canvas.create_oval(x * self.width + 2, y * self.width + 2, (x + 1) * self.width - 2, (y + 1) * self.width - 2, fill='white')
def restart(self):
self.current_player = self.start
self.chessboard = [[0] * self.size for _ in range(self.size)]
self.canvas.delete('all')
self.create_widgets()
if __name__ == '__main__':
root = tk.Tk()
root.title('Five in a Row')
game = Chessboard(root)
root.mainloop()
```
运行该代码后,会弹出一个窗口,可以在其中进行五子棋游戏。用户可以选择先手或后手。当游戏结束时,会显示胜利者或平局。用户可以点击Restart按钮重新开始游戏。
阅读全文
相关推荐

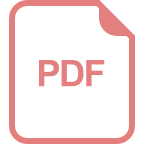
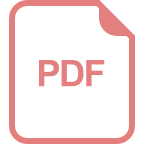
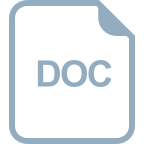
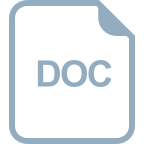
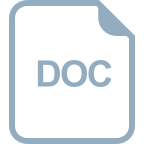
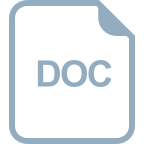
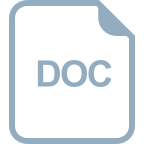
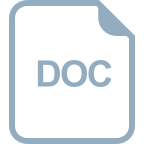
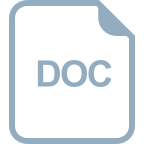
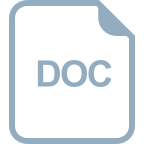
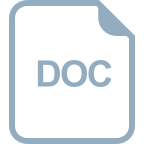
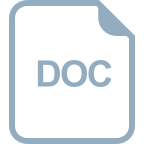
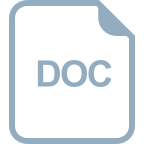
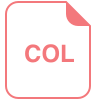
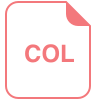