用python设计一款五子棋游戏,首先,五子棋游戏的规则是两个玩家轮流在棋盘上下黑白两种颜色的棋子,先在横、竖、斜线上形成连续的五个同色棋子者获胜。
时间: 2024-03-24 10:40:55 浏览: 16
好的,这里给您提供一份基于Python语言实现的五子棋游戏代码,希望能帮助您。
```Python
import pygame
import sys
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
size = width, height = 600, 600
# 设置颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GREY = (211, 211, 211)
# 设置棋盘大小
BOARD_SIZE = 15
# 设置棋盘格子大小
CELL_SIZE = 40
# 设置棋子半径
RADIUS = 18
# 设置棋子边缘大小
BORDER_SIZE = 2
# 设置棋子颜色
BLACK_STONE = pygame.image.load('black.png')
WHITE_STONE = pygame.image.load('white.png')
# 初始化棋盘矩阵
board = [[0] * BOARD_SIZE for i in range(BOARD_SIZE)]
# 初始化屏幕
screen = pygame.display.set_mode(size)
# 绘制棋盘
def draw_board():
for i in range(BOARD_SIZE):
# 绘制横线
pygame.draw.line(screen, BLACK, (CELL_SIZE, (i + 1) * CELL_SIZE), ((BOARD_SIZE - 1) * CELL_SIZE, (i + 1) * CELL_SIZE), BORDER_SIZE)
# 绘制竖线
pygame.draw.line(screen, BLACK, ((i + 1) * CELL_SIZE, CELL_SIZE), ((i + 1) * CELL_SIZE, (BOARD_SIZE - 1) * CELL_SIZE), BORDER_SIZE)
# 绘制棋子
def draw_stone(x, y, color):
if color == 'black':
screen.blit(BLACK_STONE, (x - RADIUS, y - RADIUS))
else:
screen.blit(WHITE_STONE, (x - RADIUS, y - RADIUS))
# 判断棋子是否在棋盘内
def is_in_board(x, y):
if x >= CELL_SIZE and x <= (BOARD_SIZE - 1) * CELL_SIZE and y >= CELL_SIZE and y <= (BOARD_SIZE - 1) * CELL_SIZE:
return True
else:
return False
# 获取鼠标点击的位置
def get_clicked_pos(x, y):
col = int(round((x - CELL_SIZE) / CELL_SIZE))
row = int(round((y - CELL_SIZE) / CELL_SIZE))
return row, col
# 判断某个位置是否有棋子
def is_empty(row, col):
if board[row][col] == 0:
return True
else:
return False
# 判断是否有五个连续的同色棋子
def has_five_in_line(row, col, color):
count = 0
# 判断横向是否有五个同色棋子
for i in range(max(0, col - 4), min(col + 1, BOARD_SIZE - 4)):
if board[row][i] == color and board[row][i+1] == color and board[row][i+2] == color and board[row][i+3] == color and board[row][i+4] == color:
return True
# 判断纵向是否有五个同色棋子
for i in range(max(0, row - 4), min(row + 1, BOARD_SIZE - 4)):
if board[i][col] == color and board[i+1][col] == color and board[i+2][col] == color and board[i+3][col] == color and board[i+4][col] == color:
return True
# 判断左上到右下是否有五个同色棋子
for i in range(max(0, row - 4), min(row + 1, BOARD_SIZE - 4)):
for j in range(max(0, col - 4), min(col + 1, BOARD_SIZE - 4)):
if board[i][j] == color and board[i+1][j+1] == color and board[i+2][j+2] == color and board[i+3][j+3] == color and board[i+4][j+4] == color:
return True
# 判断左下到右上是否有五个同色棋子
for i in range(max(0, row - 4), min(row + 1, BOARD_SIZE - 4)):
for j in range(min(col + 4, BOARD_SIZE - 1), max(col - 1, 3), -1):
if board[i][j] == color and board[i+1][j-1] == color and board[i+2][j-2] == color and board[i+3][j-3] == color and board[i+4][j-4] == color:
return True
return False
# 判断游戏是否结束
def is_game_over(color):
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == 0:
if has_five_in_line(i, j, color):
return True
return False
# 初始化游戏
def init_game():
global board
board = [[0] * BOARD_SIZE for i in range(BOARD_SIZE)]
draw_board()
pygame.display.update()
# 人机对弈
def play_game():
# 人机对弈,黑棋先手
color = 'black'
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:
x, y = event.pos
if not is_in_board(x, y):
continue
row, col = get_clicked_pos(x, y)
if not is_empty(row, col):
continue
draw_stone((col + 1) * CELL_SIZE, (row + 1) * CELL_SIZE, color)
board[row][col] = color
if is_game_over(color):
print(color + ' wins!')
init_game()
if color == 'black':
color = 'white'
else:
color = 'black'
pygame.display.update()
# 执行游戏
init_game()
play_game()
# 退出Pygame
pygame.quit()
```
该代码实现了基本的五子棋游戏规则,同时支持人机对弈,具有较好的可玩性。其中,棋盘的绘制、棋子的绘制、鼠标点击位置的获取、棋子是否在棋盘内、棋子是否重叠、是否有五个连续的同色棋子等功能均已实现。
需要注意的是,该代码仅为基础实现,还有很多细节和优化可以进行。比如,可以添加背景音乐、游戏结束后可以显示胜利者的信息等等。
相关推荐
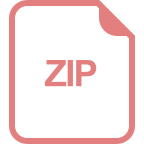
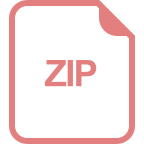
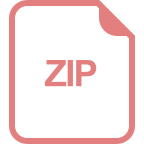














