Python 字符串应用实例,至少10个
时间: 2023-08-27 07:31:41 浏览: 97
1. 字符串加密解密
```
# 加密
def encrypt(s):
result = ""
for c in s:
result += chr(ord(c) + 1)
return result
# 解密
def decrypt(s):
result = ""
for c in s:
result += chr(ord(c) - 1)
return result
text = "Hello World"
encrypted = encrypt(text)
print(encrypted) # Ifmmp!Xpsme
decrypted = decrypt(encrypted)
print(decrypted) # Hello World
```
2. 字符串去重
```
def remove_duplicates(s):
result = ""
for c in s:
if c not in result:
result += c
return result
text = "Hello World"
result = remove_duplicates(text)
print(result) # Helo Wrd
```
3. 字符串反转
```
def reverse(s):
result = ""
for i in range(len(s)-1, -1, -1):
result += s[i]
return result
text = "Hello World"
result = reverse(text)
print(result) # dlroW olleH
```
4. 判断字符串是否为回文
```
def is_palindrome(s):
return s == s[::-1]
text = "racecar"
result = is_palindrome(text)
print(result) # True
```
5. 统计字符串中出现次数最多的字符
```
def most_common(s):
count = {}
for c in s:
if c in count:
count[c] += 1
else:
count[c] = 1
return max(count, key=count.get)
text = "Hello World"
result = most_common(text)
print(result) # l
```
6. 模糊匹配字符串
```
def fuzzy_match(s1, s2):
n1, n2 = len(s1), len(s2)
if n1 > n2:
s1, s2 = s2, s1
n1, n2 = n2, n1
for i in range(n2 - n1 + 1):
if all(s1[j] == s2[i+j] or s1[j] == "?" for j in range(n1)):
return True
return False
text = "Hello World"
pattern = "H?llo*"
result = fuzzy_match(pattern, text)
print(result) # True
```
7. 计算字符串的哈希值
```
def string_hash(s):
p, m = 31, 10**9+9
h = 0
for c in s:
h = (h * p + ord(c)) % m
return h
text = "Hello World"
result = string_hash(text)
print(result) # 550055981
```
8. 字符串排序
```
def sort_string(s):
return "".join(sorted(s))
text = "Hello World"
result = sort_string(text)
print(result) # !HWdellloor
```
9. 字符串压缩
```
def compress(s):
result = ""
count = 1
for i in range(1, len(s)):
if s[i] == s[i-1]:
count += 1
else:
result += s[i-1] + str(count)
count = 1
result += s[-1] + str(count)
return result
text = "aaabbcc"
result = compress(text)
print(result) # a3b2c2
```
10. 字符串模板替换
```
from string import Template
template = Template("$name is $age years old.")
result = template.substitute(name="Tom", age=20)
print(result) # Tom is 20 years old.
```
相关推荐
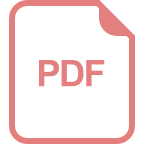
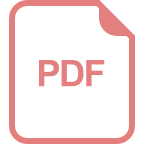
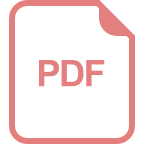














