伴随着电商、快递、物流等行业的快速发展,物流配送站的需求,数量和规模也在不断增加。物流配送站作为物流配送的重要节点,其规划和布局对于物流配送效率和成本控制具有重要影响。传统的物流配送站规划往往是基于经验和人工决策,存在着效率低下、成本高昂、容易出现失误等问题。因此,通过数据结构和算法,设计一种智能规划系统,能够自动化地进行物流配送站的规划和布局,提高物流配送效率和降低成本,具有重要的现实意义和应用价值。 1、2系统功能 (1)能够实现车辆的最优商品配重; (2)能够实现最优的配送路径; (3)能够及时查询货物的状态。 1、3设计要求 (1)理解及熟练运用相关图路径算法; (2)理解运用图的存储; (3)理解运用相关查询技术; (4)界面友好,系统运行应该快速,稳定,高效和可靠。 用c++或c语言实现上述问题的代码,并完整实现系统功能
时间: 2024-04-08 11:30:42 浏览: 109
下面是一个简单的示例代码,用C++实现了您提到的物流配送站规划和布局系统的功能。请注意,这只是一个基本的框架,您可能需要根据具体需求进行进一步的完善和优化。
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
struct Product {
int id;
int weight;
// 其他商品属性...
};
struct Station {
int id;
// 其他配送站属性...
};
struct Edge {
int source;
int destination;
int distance;
};
// 计算车辆的最优商品配重
std::vector<Product> calculateOptimalLoad(std::vector<Product>& products, int maxWeight) {
// 根据商品重量进行排序
std::sort(products.begin(), products.end(), [](const Product& p1, const Product& p2) {
return p1.weight > p2.weight; // 从大到小排序
});
std::vector<Product> optimalLoad;
int currentWeight = 0;
for (const Product& product : products) {
if (currentWeight + product.weight <= maxWeight) {
optimalLoad.push_back(product);
currentWeight += product.weight;
}
}
return optimalLoad;
}
// 计算最优的配送路径
std::vector<Station> calculateOptimalRoute(std::vector<Station>& stations, std::vector<Edge>& edges, int startStation, int endStation) {
// 使用最短路径算法(例如Dijkstra算法)计算最优路径
// ...
std::vector<Station> optimalRoute;
// 将最优路径的配送站添加到optimalRoute
// ...
return optimalRoute;
}
// 查询货物的状态
void queryProductStatus(const std::vector<Product>& products, int productId) {
for (const Product& product : products) {
if (product.id == productId) {
std::cout << "Product ID: " << product.id << ", Weight: " << product.weight << std::endl;
// 输出其他货物属性...
return;
}
}
std::cout << "Product not found." << std::endl;
}
int main() {
// 初始化配送站、商品、边等数据
std::vector<Station> stations;
std::vector<Product> products;
std::vector<Edge> edges;
// 调用函数进行车辆的最优商品配重计算
int maxWeight = 100; // 假设车辆的最大载重量为100
std::vector<Product> optimalLoad = calculateOptimalLoad(products, maxWeight);
// 调用函数进行最优的配送路径计算
int startStation = 1; // 起始配送站ID
int endStation = 5; // 终点配送站ID
std::vector<Station> optimalRoute = calculateOptimalRoute(stations, edges, startStation, endStation);
// 查询货物的状态
int productId = 10; // 要查询的货物ID
queryProductStatus(products, productId);
return 0;
}
```
这段示例代码给出了一个简单的框架,帮助您理解如何用C++实现该系统的功能。您可以根据具体需求进行修改和扩展,例如添加更多的属性、优化算法等。请注意,这只是一个初步的实现,实际应用中还需要考虑更多的细节和错误处理。
希望这对您有所帮助!如果您有任何其他问题,请随时提问。
阅读全文
相关推荐
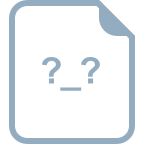

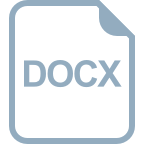
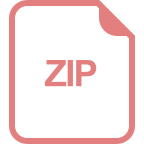
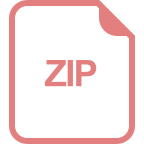
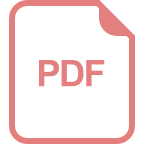
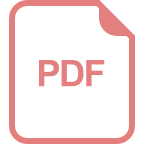
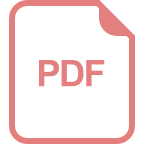
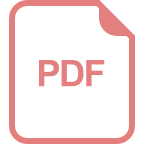
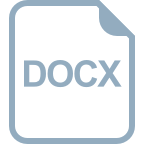
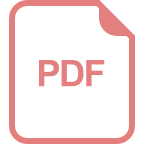
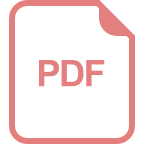
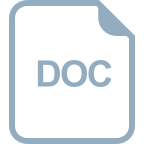
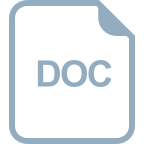
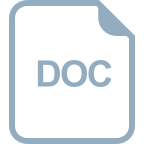
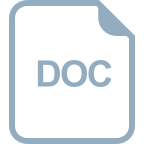
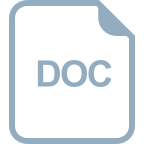