定义描述职工信息的结构体变量,编写C语言程序实现n个员工数据信息的输入和输出功能,数据信息结构:工号,姓名,性别,年龄,工龄,工资和家庭住址
时间: 2023-09-25 15:10:07 浏览: 64
以下是定义结构体变量和实现输入输出功能的 C 代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_EMPLOYEES 50
struct Employee {
int id;
char name[50];
char gender;
int age;
int years_of_service;
float salary;
char address[100];
};
int main() {
struct Employee employees[MAX_EMPLOYEES];
int n;
printf("请输入员工人数(不超过 %d 人):", MAX_EMPLOYEES);
scanf("%d", &n);
// 输入员工数据信息
for (int i = 0; i < n; i++) {
printf("请输入第 %d 个员工的信息:\n", i + 1);
printf("工号:");
scanf("%d", &employees[i].id);
printf("姓名:");
scanf("%s", employees[i].name);
printf("性别:");
scanf(" %c", &employees[i].gender);
printf("年龄:");
scanf("%d", &employees[i].age);
printf("工龄:");
scanf("%d", &employees[i].years_of_service);
printf("工资:");
scanf("%f", &employees[i].salary);
printf("家庭住址:");
scanf("%s", employees[i].address);
}
// 输出员工数据信息
printf("员工数据信息如下:\n");
printf("工号\t姓名\t性别\t年龄\t工龄\t工资\t家庭住址\n");
for (int i = 0; i < n; i++) {
printf("%d\t%s\t%c\t%d\t%d\t%.2f\t%s\n",
employees[i].id,
employees[i].name,
employees[i].gender,
employees[i].age,
employees[i].years_of_service,
employees[i].salary,
employees[i].address
);
}
return 0;
}
```
程序运行时会要求输入员工人数,然后输入每个员工的信息,最后输出所有员工的数据信息。
相关推荐
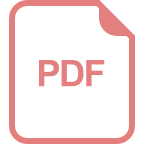
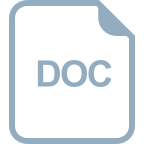














