static inline double getYaw(const geometry_msgs::Quaternion& msg_q){ Quaternion bt_q; quaternionMsgToTF(msg_q, bt_q); return getYaw(bt_q); }
时间: 2024-02-05 14:13:56 浏览: 219
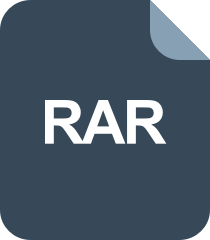
msg.rar_linux 消息队列_linux msgrcv_msg_消息队列
这是一个 C++ 函数,用于从 ROS 消息类型的 geometry_msgs::Quaternion 中获取航向角(yaw)。具体实现步骤如下:
1. 将 geometry_msgs::Quaternion 消息类型转换为 tf::Quaternion(Transform Library 中定义的四元数类型)。
2. 使用一个名为 getYaw 的函数从 tf::Quaternion 中获取航向角。
3. 将航向角作为 double 类型返回。
其中,quaternionMsgToTF 是一个 ROS 提供的函数,用于将 geometry_msgs::Quaternion 转换为 tf::Quaternion。getYaw 函数的实现可以参考以下代码:
```
double getYaw(const tf::Quaternion& q)
{
double roll, pitch, yaw;
tf::Matrix3x3(q).getRPY(roll, pitch, yaw);
return yaw;
}
```
这个函数使用了 tf::Matrix3x3 类型的 getRPY 函数,从四元数中获取欧拉角的 roll、pitch 和 yaw。由于我们只需要获取 yaw,因此这里直接返回 yaw 值即可。
阅读全文
相关推荐
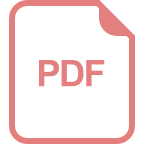
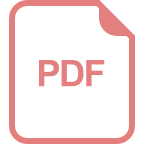





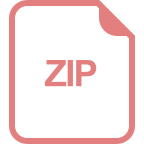
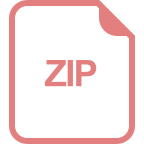
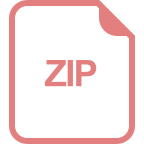
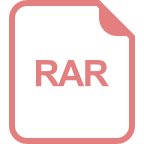
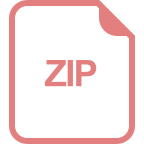
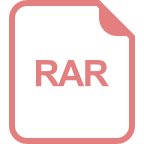