python写一颗会动的圣诞树
时间: 2023-02-13 17:52:13 浏览: 121
好的,下面是一个简单的例子,使用 Python 的 turtle 库来绘制一颗会动的圣诞树:
```
import turtle
import time
def draw_tree(t, branch_len):
if branch_len > 5:
t.forward(branch_len)
t.right(20)
draw_tree(t, branch_len-15)
t.left(40)
draw_tree(t, branch_len-15)
t.right(20)
t.backward(branch_len)
def animate_tree(t):
for i in range(10):
t.right(5)
draw_tree(t, 75)
time.sleep(0.1)
t = turtle.Turtle()
t.left(90)
t.up()
t.backward(100)
t.down()
t.color("green")
animate_tree(t)
turtle.done()
```
这个程序会在屏幕上绘制一棵动态的圣诞树,每隔 0.1 秒会有轻微的角度变化。
如果你对 turtle 库不熟悉,可以先学习一下基础用法。
相关问题
python写一颗会动的彩色的圣诞树
你好!我可以为你提供一个简单的 Python 代码来实现彩色圣诞树的效果,你可以使用这个代码作为参考来自己实现:
```python
import time
colors = ['red', 'green', 'yellow', 'blue', 'purple']
def show_tree(colors):
for color in colors:
print(f" * {color}")
print(f" *** {color}")
print(f" ***** {color}")
print(f"*******{color}")
while True:
show_tree(colors)
time.sleep(1)
```
上述代码会循环展示一棵圣诞树,每一秒钟更换一次树的颜色。你可以自己定义树的形状和颜色,并使用 `time.sleep()` 函数来控制展示速度。
希望这些信息能帮到你!
用python画一颗会动的圣诞树
当然可以!
为了画出一颗会动的圣诞树,我们可以使用 Python 的绘图库 `matplotlib`。
首先,我们需要导入 `matplotlib` 库:
```python
import matplotlib.pyplot as plt
```
然后,我们可以使用 `plt.plot` 函数来绘制圣诞树的轮廓线。例如,下面的代码可以绘制出一颗三角形的圣诞树:
```python
plt.plot([0, 0.5, 1], [0, 1, 0])
```
为了让圣诞树看起来更加真实,我们可以给圣诞树添加一些装饰,例如圣诞球。这可以通过使用 `plt.scatter` 函数来实现:
```python
plt.scatter([0.25, 0.75], [0.5, 0.5], c='red', s=50)
```
接下来,我们可以使用 `plt.show` 函数来显示圣诞树:
```python
plt.show()
```
如果想要让圣诞树动起来,我们可以使用 Python 的动画库 `matplotlib.animation`。
首先,我们需要导入 `matplotlib.animation` 库:
```python
import matplotlib.animation as animation
```
然后,我们可以定义一个函数,该函数负责绘制圣诞树的当前状态。例如,下面的函数可以让圣诞树摇摆:
```python
def update(num):
x = [0, 0.5 + 0.1 * np.sin(num), 1]
y = [0, 1 + 0.1 * np.sin(num), 0]
plt.plot(x, y)
plt.scatter([0.25
阅读全文
相关推荐
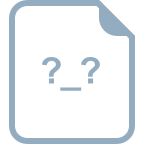
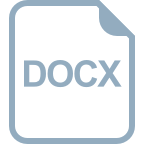






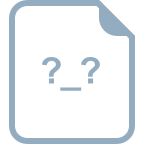
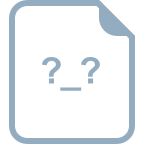