用java编写一个上传下载代码示例
时间: 2023-08-24 13:09:30 浏览: 147
### 回答1:
下面是一个用 Java 编写的上传文件的示例代码:
```
import java.io.*;
import java.net.*;
import java.util.*;
public class FileUpload {
public static void main(String[] args) throws Exception {
String fileName = "test.txt";
String urlString = "http://www.example.com/upload";
// Create a URL object
URL url = new URL(urlString);
// Open a connection to the URL
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// Set the request method to "POST"
connection.setRequestMethod("POST");
// Set the request properties
connection.setRequestProperty("Content-Type", "multipart/form-data");
connection.setRequestProperty("Content-Length", String.valueOf(new File(fileName).length()));
// Enable output
connection.setDoOutput(true);
// Open a output stream to the server
DataOutputStream outputStream = new DataOutputStream(connection.getOutputStream());
// Open an input stream to the file
FileInputStream inputStream = new FileInputStream(fileName);
// Read the contents of the file into a byte array
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
// Write the bytes to the output stream
outputStream.write(buffer, 0, bytesRead);
}
// Close the input and output streams
inputStream.close();
outputStream.close();
// Get the response code from the server
int responseCode = connection.getResponseCode();
System.out.println("Response code: " + responseCode);
// Read the response from the server
BufferedReader reader = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
}
}
```
下面是一个用 Java 编写的下载文件的示例代码:
```
import java.io.*;
import java.net.*;
public class FileDownload {
public static void main(String[] args) throws Exception {
String fileUrl = "http://www.example.com/download/test.txt";
String fileName = "test.txt";
// Open a connection to the URL
URL url = new URL(fileUrl);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// Set the request method to "GET"
connection.setRequestMethod("GET");
// Open an input stream to the file
InputStream inputStream = connection.getInputStream();
// Create a file output stream
FileOutputStream outputStream = new FileOutputStream(fileName);
// Read the contents of the file into a byte array
byte[] buffer = new byte[1024];
int
### 回答2:
下面是一个使用Java编写的简单的上传和下载代码示例:
上传代码示例:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class UploadExample {
public static void main(String[] args) {
String fileUrl = "路径/文件名"; // 文件路径和名称
String uploadUrl = "上传目标URL"; // 上传目标的URL
try {
File file = new File(fileUrl);
URL url = new URL(uploadUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoOutput(true);
conn.setDoInput(true);
conn.setRequestMethod("POST");
conn.setRequestProperty("Content-Type", "multipart/form-data;boundary=" + "*****");
OutputStream outputStream = conn.getOutputStream();
FileInputStream fileInputStream = new FileInputStream(file);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fileInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
fileInputStream.close();
outputStream.flush();
outputStream.close();
InputStream inputStream = conn.getInputStream();
// 处理上传结果
inputStream.close();
conn.disconnect();
System.out.println("文件上传成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
下载代码示例:
```java
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
public class DownloadExample {
public static void main(String[] args) {
String downloadUrl = "下载文件URL"; // 下载文件的URL
String savePath = "保存路径/文件名"; // 下载文件保存路径和名称
try {
URL url = new URL(downloadUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
InputStream inputStream = conn.getInputStream();
byte[] buffer = new byte[1024];
OutputStream outputStream = new FileOutputStream(savePath);
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
inputStream.close();
outputStream.flush();
outputStream.close();
conn.disconnect();
System.out.println("文件下载成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
以上示例代码可以实现简单的上传和下载功能,具体使用时,需要修改路径、URL等相关参数来适应实际的应用场景。
### 回答3:
以下是一个使用Java编写的上传下载代码示例:
1.上传文件:
```
import java.io.*;
import java.net.*;
public class FileUploader {
public static void main(String[] args) {
try {
File file = new File("path/to/file"); // 要上传的文件路径
String url = "http://localhost/upload.php"; // 上传文件的URL
HttpURLConnection connection = (HttpURLConnection) new URL(url).openConnection();
connection.setDoOutput(true);
connection.setRequestMethod("POST");
OutputStream outputStream = connection.getOutputStream();
FileInputStream fileInputStream = new FileInputStream(file);
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = fileInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
outputStream.close();
fileInputStream.close();
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// 文件上传成功
System.out.println("文件上传成功");
} else {
// 文件上传失败
System.out.println("文件上传失败");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
2.下载文件:
```
import java.io.*;
import java.net.*;
public class FileDownloader {
public static void main(String[] args) {
try {
String fileUrl = "http://localhost/files/sample.txt"; // 要下载的文件URL
String savePath = "path/to/save"; // 保存文件的路径
HttpURLConnection connection = (HttpURLConnection) new URL(fileUrl).openConnection();
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
InputStream inputStream = connection.getInputStream();
FileOutputStream fileOutputStream = new FileOutputStream(savePath);
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = inputStream.read(buffer)) != -1) {
fileOutputStream.write(buffer, 0, bytesRead);
}
fileOutputStream.close();
inputStream.close();
// 文件下载成功
System.out.println("文件下载成功");
} else {
// 文件下载失败
System.out.println("文件下载失败");
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
注意:上述示例中的URL和文件路径需要根据实际情况进行修改。上传文件示例中的`upload.php`可以是后端处理上传文件的接口,也可以是其他符合需求的URL。下载文件示例中的`sample.txt`是要下载的文件名,也可以是其他文件名。
阅读全文
相关推荐
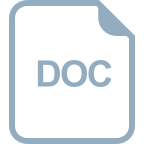
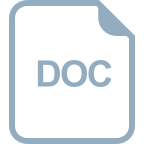
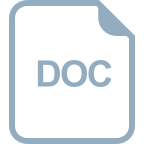
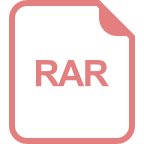
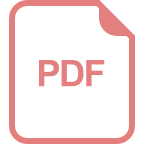
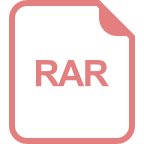
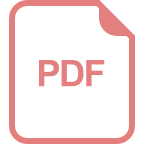
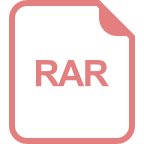
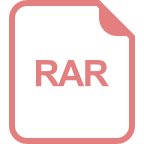
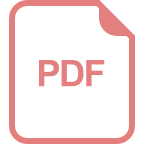
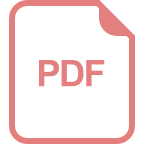
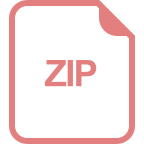






