aes加密算法c语言实现
时间: 2023-07-13 07:22:36 浏览: 65
以下是使用 OpenSSL 库进行 AES 加密和解密的示例 C 代码:
```c
#include <openssl/aes.h>
#include <string.h>
void encrypt(unsigned char *plaintext, int plaintext_len, unsigned char *key,
unsigned char *iv, unsigned char *ciphertext) {
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_cbc_encrypt(plaintext, ciphertext, plaintext_len, &aes_key, iv, AES_ENCRYPT);
}
void decrypt(unsigned char *ciphertext, int ciphertext_len, unsigned char *key,
unsigned char *iv, unsigned char *plaintext) {
AES_KEY aes_key;
AES_set_decrypt_key(key, 128, &aes_key);
AES_cbc_encrypt(ciphertext, plaintext, ciphertext_len, &aes_key, iv, AES_DECRYPT);
}
int main() {
unsigned char *key = (unsigned char *)"0123456789abcdef"; // 128-bit key
unsigned char *iv = (unsigned char *)"abcdef0123456789"; // 128-bit IV
unsigned char plaintext[] = "Hello, world!";
int plaintext_len = strlen((char *)plaintext);
// Determine the required size of the ciphertext buffer
int ciphertext_len = ((plaintext_len - 1) / AES_BLOCK_SIZE + 1) * AES_BLOCK_SIZE;
// Allocate memory for the ciphertext buffer
unsigned char *ciphertext = malloc(ciphertext_len);
// Encrypt the plaintext
encrypt(plaintext, plaintext_len, key, iv, ciphertext);
// Print the ciphertext
printf("Ciphertext: ");
for (int i = 0; i < ciphertext_len; i++) {
printf("%02x", ciphertext[i]);
}
printf("\n");
// Allocate memory for the decrypted plaintext buffer
unsigned char *decrypted_plaintext = malloc(ciphertext_len);
// Decrypt the ciphertext
decrypt(ciphertext, ciphertext_len, key, iv, decrypted_plaintext);
// Print the decrypted plaintext
printf("Decrypted plaintext: %s\n", decrypted_plaintext);
// Free memory
free(ciphertext);
free(decrypted_plaintext);
return 0;
}
```
在上述代码中,我们使用了 OpenSSL 库的 AES 函数来实现加密和解密功能。其中,`AES_set_encrypt_key` 和 `AES_set_decrypt_key` 函数用于设置加密和解密所需的密钥,`AES_cbc_encrypt` 函数用于实现 CBC 模式的加密和解密。在主函数中,我们首先定义了一个 128 位的密钥和 IV,然后分别对明文进行加密和解密,并输出加密后的密文和解密后的明文。需要注意的是,为了方便输出,我们在代码中使用了 `printf` 函数,如果在实际应用中需要保证加密后的密文不被修改,应该使用更安全的输出方式,如将密文写入文件或发送到网络中。
相关推荐
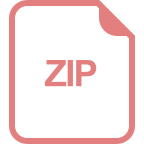
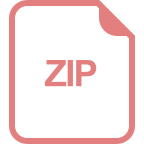














