ptqt5 自定义标题栏
时间: 2023-12-26 12:49:24 浏览: 130
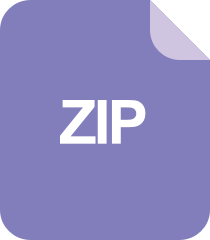
qt5 自定义标题栏

使用Qt Widgets模块中的QWidget和QHBoxLayout类,可以自定义一个具有自定义标题栏的窗口。
以下是创建自定义标题栏的步骤:
1. 创建QWidget对象,用于作为窗口的标题栏。
2. 创建QHBoxLayout对象,并将其设置为QWidget的布局管理器。
3. 创建QLabel对象,并将其设置为QWidget的子控件,用于显示窗口的标题。
4. 创建QPushButton对象,并将其设置为QWidget的子控件,用于关闭窗口。
5. 重写QWidget的mousePressEvent和mouseMoveEvent方法,以实现窗口的移动。
下面是一个示例代码:
```python
from PyQt5.QtWidgets import QWidget, QHBoxLayout, QLabel, QPushButton
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import Qt
class CustomTitleBar(QWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.initUI()
def initUI(self):
# 设置窗口标题栏的背景色和高度
self.setStyleSheet("background-color:gray;")
self.setFixedHeight(30)
# 创建标题和关闭按钮
self.titleLabel = QLabel("Custom Title Bar")
self.closeButton = QPushButton(QIcon("close.png"), "")
self.closeButton.setFixedSize(16, 16)
self.closeButton.clicked.connect(self.parent().close)
# 创建水平布局管理器,并将标题和关闭按钮添加到布局中
layout = QHBoxLayout(self)
layout.addWidget(self.titleLabel)
layout.addStretch()
layout.addWidget(self.closeButton)
def mousePressEvent(self, event):
# 记录当前鼠标位置和窗口位置差
self.offset = event.pos()
def mouseMoveEvent(self, event):
# 移动窗口
x = event.globalX()
y = event.globalY()
self.parent().move(x - self.offset.x(), y - self.offset.y())
```
在主窗口中,创建CustomTitleBar对象,并将其设置为窗口的标题栏:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow
import sys
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 设置窗口标题和大小
self.setWindowTitle("Custom Title Bar Example")
self.setGeometry(100, 100, 400, 300)
# 创建自定义标题栏
self.titleBar = CustomTitleBar(self)
self.setMenuWidget(self.titleBar)
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
运行程序后,即可看到具有自定义标题栏的窗口。用户可以通过点击窗口的标题栏来移动窗口,也可以通过点击窗口的关闭按钮来关闭窗口。
阅读全文
相关推荐
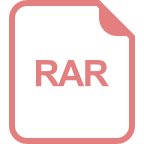
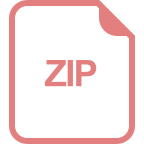
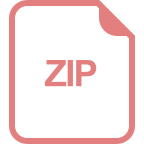
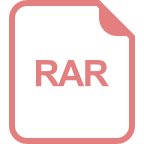
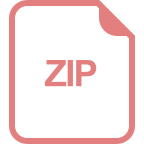
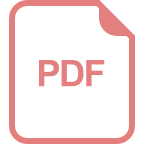
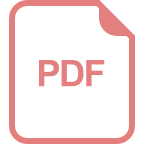
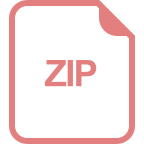
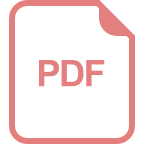
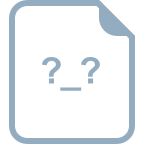
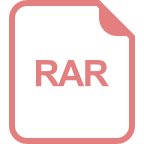





