python tf.Graph
时间: 2023-06-18 10:07:20 浏览: 51
在TensorFlow中,图(Graph)是一个计算模型,它由节点(Node)和边(Edge)组成。节点表示操作(Operation),边表示数据流(Tensor)。在TensorFlow中,我们首先定义一个图,然后再在图中定义节点和边。
在Python中,我们可以使用tf.Graph()方法创建一个图,并使用with tf.Session(graph=g)方法将计算图传递给会话(Session)来执行计算。这种方式可以帮助我们在同一程序中使用多个计算图,同时还可以避免不同的计算图之间发生冲突。
例如,以下代码演示了如何创建一个简单的计算图并使用会话运行它:
```
import tensorflow as tf
# 创建一个计算图
graph = tf.Graph()
# 在计算图中定义节点和边
with graph.as_default():
a = tf.constant(2)
b = tf.constant(3)
c = a + b
# 使用会话执行计算图
with tf.Session(graph=graph) as sess:
result = sess.run(c)
print(result)
```
在上面的示例中,我们创建了一个计算图,其中包含两个常量节点和一个加法节点。然后,我们使用会话运行计算图,并得到结果5。
相关问题
tf.Graph().as_default():
`tf.Graph().as_default()`是TensorFlow中的一个上下文管理器,它用于指定一个默认的计算图(graph)。TensorFlow程序中的计算图是由一系列的操作(`tf.Operation`)和张量(`tf.Tensor`)构成的。在TensorFlow中,默认情况下会自动创建一个默认的计算图,可以通过`tf.get_default_graph()`访问。
在使用TensorFlow时,如果需要定义多个计算图,可以使用`tf.Graph()`创建新的计算图,并使用`as_default()`方法将其设置为默认的计算图,这样在新的计算图中定义的操作和张量就会被添加到该计算图中。例如:
```python
import tensorflow as tf
graph1 = tf.Graph()
with graph1.as_default():
# 在graph1中定义操作和张量
a = tf.constant(1)
b = tf.constant(2)
c = a + b
graph2 = tf.Graph()
with graph2.as_default():
# 在graph2中定义操作和张量
x = tf.constant(3)
y = tf.constant(4)
z = x * y
with tf.Session(graph=graph1) as sess1:
# 在sess1中运行graph1中定义的操作
result1 = sess1.run(c)
with tf.Session(graph=graph2) as sess2:
# 在sess2中运行graph2中定义的操作
result2 = sess2.run(z)
```
在上面的例子中,我们创建了两个计算图`graph1`和`graph2`,并在每个计算图中定义了一些操作和张量。然后,我们在不同的会话(`tf.Session`)中分别运行了这些操作,并得到了不同的结果。
with tf.Graph().as_default(), tf.Session() as sess: AttributeError: module 'tensorflow' has no attribute 'Session'
在 TensorFlow 2.x 版本中,`tf.Session()` 已经被废弃,而被 `tf.compat.v1.Session()` 取代。如果你的代码中使用了 `tf.Session()`,可以改为 `tf.compat.v1.Session()`,或者使用 `tf.compat.v1.disable_v2_behavior()` 函数来启用 TensorFlow 1.x 的行为。示例代码如下:
```python
import tensorflow.compat.v1 as tf
tf.disable_v2_behavior()
with tf.Graph().as_default(), tf.Session() as sess:
# 在 TensorFlow 1.x 中使用 Session
```
如果你的 TensorFlow 版本是 1.x,可以直接使用 `tf.Session()`。
相关推荐
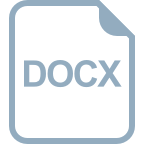
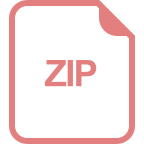
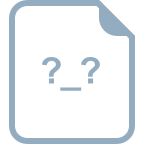












