使用C语言设计一段程序,输入50个学生的记录,每个学生的记录包含学号和成绩,组成记录数组,然后按照成绩由高到低的次序输出,排序方式采用快速排序
时间: 2023-09-25 09:06:49 浏览: 133
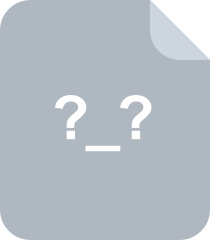
课程设计,用的是c语言,可以实现简单的成绩记录
### 回答1:
好的,以下是使用C语言设计的程序代码:
#include <stdio.h>
// 定义学生记录结构体类型
typedef struct {
int id;
int score;
} StudentRecord;
// 快速排序函数
void quickSort(StudentRecord records[], int left, int right) {
if (left >= right) {
return;
}
int i = left;
int j = right;
StudentRecord pivot = records[left];
while (i < j) {
while (i < j && records[j].score <= pivot.score) {
j--;
}
records[i] = records[j];
while (i < j && records[i].score >= pivot.score) {
i++;
}
records[j] = records[i];
}
records[i] = pivot;
quickSort(records, left, i - 1);
quickSort(records, i + 1, right);
}
int main() {
// 定义学生记录数组
StudentRecord records[50];
// 输入学生记录
int i;
for (i = 0; i < 50; i++) {
printf("请输入第%d个学生的学号和成绩,用空格分隔:", i + 1);
scanf("%d%d", &records[i].id, &records[i].score);
}
// 对学生记录进行排序
quickSort(records, 0, 49);
// 按照成绩由高到低的次序输出学生记录
printf("--------------------\n");
printf("按照成绩由高到低的次序输出学生记录:\n");
for (i = 0; i < 50; i++) {
printf("%d %d\n", records[i].id, records[i].score);
}
return 0;
}
### 回答2:
以下是使用C语言设计的满足要求的程序:
```c
#include <stdio.h>
typedef struct {
int studentID;
float score;
} StudentRecord;
void swap(StudentRecord* a, StudentRecord* b) {
StudentRecord temp = *a;
*a = *b;
*b = temp;
}
int partition(StudentRecord records[], int low, int high) {
float pivot = records[high].score;
int i = low - 1;
for (int j = low; j < high; j++) {
if (records[j].score >= pivot) {
i++;
swap(&records[i], &records[j]);
}
}
swap(&records[i + 1], &records[high]);
return i + 1;
}
void quickSort(StudentRecord records[], int low, int high) {
if (low < high) {
int pivotIndex = partition(records, low, high);
quickSort(records, low, pivotIndex - 1);
quickSort(records, pivotIndex + 1, high);
}
}
int main() {
int numStudents = 50;
StudentRecord records[numStudents];
// 输入学生记录
for (int i = 0; i < numStudents; i++) {
printf("请输入第%d个学生的学号和成绩:", i + 1);
scanf("%d%f", &records[i].studentID, &records[i].score);
}
// 使用快速排序按成绩由高到低排序
quickSort(records, 0, numStudents - 1);
// 输出排序好的学生记录
printf("\n按成绩由高到低的次序输出学生记录:\n");
for (int i = 0; i < numStudents; i++) {
printf("学号:%d,成绩:%f\n", records[i].studentID, records[i].score);
}
return 0;
}
```
以上程序通过定义`StudentRecord`结构体来存储学生的学号和成绩。然后使用`partition`函数和`quickSort`函数来实现快速排序。在主函数中,先输入50个学生的学号和成绩,然后使用快速排序按照成绩由高到低对学生记录进行排序,最后输出排序好的学生记录。
### 回答3:
以下是使用C语言设计的程序:
```c
#include <stdio.h>
typedef struct {
int id;
float score;
} Student;
void swap(Student* a, Student* b) {
Student temp = *a;
*a = *b;
*b = temp;
}
int partition(Student arr[], int low, int high) {
float pivot = arr[high].score;
int i = low - 1;
for (int j = low; j < high; j++) {
if (arr[j].score >= pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return i + 1;
}
void quickSort(Student arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
int main() {
int numStudents = 50;
Student students[numStudents];
// 输入学生记录
for (int i = 0; i < numStudents; i++) {
printf("请输入第%d个学生的学号:", i + 1);
scanf("%d", &students[i].id);
printf("请输入第%d个学生的成绩:", i + 1);
scanf("%f", &students[i].score);
}
quickSort(students, 0, numStudents - 1);
// 输出排序后的结果
printf("按照成绩由高到低排序后的结果:\n");
for (int i = 0; i < numStudents; i++) {
printf("学号:%d,成绩:%.2f\n", students[i].id, students[i].score);
}
return 0;
}
```
这段程序首先定义了一个名为`Student`的结构体,包含学生的学号和成绩。然后,定义了两个辅助函数`swap`用于交换两个学生记录的位置,`partition`用于快速排序中的划分过程。接着,定义了`quickSort`函数用于实现快速排序的递归过程。
在`main`函数中,首先定义了需要输入的学生记录的数量`numStudents`,以及一个大小为`numStudents`的学生数组`students`。然后,通过循环输入每个学生的学号和成绩。接下来,调用`quickSort`函数对学生数组进行排序。最后,使用循环按照成绩由高到低的顺序输出排序后的学生记录。
阅读全文
相关推荐
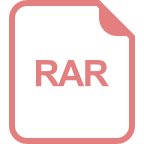


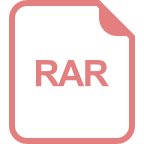












