粒子群优化算法-SVM多分类参数寻优python代码
时间: 2023-07-24 07:16:35 浏览: 66
以下是使用粒子群优化算法对SVM多分类模型参数进行寻优的Python代码:
```python
import numpy as np
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.metrics import accuracy_score
from sklearn.svm import SVC
# 定义粒子群优化算法
class PSO:
def __init__(self, num_particles, max_iter, c1, c2, w, dim):
self.num_particles = num_particles
self.max_iter = max_iter
self.c1 = c1
self.c2 = c2
self.w = w
self.dim = dim
self.x = np.random.uniform(low=-1, high=1, size=(num_particles, dim))
self.v = np.random.uniform(low=-1, high=1, size=(num_particles, dim))
self.pbest_x = self.x.copy()
self.pbest_y = np.zeros(num_particles)
self.gbest_x = np.zeros(dim)
self.gbest_y = float('inf')
def update(self, X_train, y_train):
for i in range(self.num_particles):
# 使用当前粒子位置构造支持向量机模型
clf = SVC(C=10 ** self.x[i][0], gamma=10 ** self.x[i][1], kernel='rbf')
# 训练支持向量机模型
clf.fit(X_train, y_train)
# 使用支持向量机模型预测
y_pred = clf.predict(X_train)
# 计算分类准确率
score = accuracy_score(y_train, y_pred)
# 更新个体最优解
if score > self.pbest_y[i]:
self.pbest_x[i] = self.x[i].copy()
self.pbest_y[i] = score
# 更新全局最优解
if score > self.gbest_y:
self.gbest_x = self.x[i].copy()
self.gbest_y = score
# 更新粒子速度和位置
r1 = np.random.rand(self.dim)
r2 = np.random.rand(self.dim)
self.v[i] = self.w * self.v[i] + self.c1 * r1 * (self.pbest_x[i] - self.x[i]) + self.c2 * r2 * (self.gbest_x - self.x[i])
self.x[i] = self.x[i] + self.v[i]
def fit(self, X_train, y_train):
for i in range(self.max_iter):
self.update(X_train, y_train)
# 加载鸢尾花数据集
iris = datasets.load_iris()
X = iris.data
y = iris.target
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 定义PSO算法参数
num_particles = 30
max_iter = 100
c1 = 2
c2 = 2
w = 0.8
dim = 2
# 初始化PSO算法
pso = PSO(num_particles, max_iter, c1, c2, w, dim)
# 训练PSO-SVM分类器
pso.fit(X_train, y_train)
# 使用最优参数训练支持向量机模型
clf = SVC(C=10 ** pso.gbest_x[0], gamma=10 ** pso.gbest_x[1], kernel='rbf')
clf.fit(X_train, y_train)
# 在测试集上测试模型性能
y_pred = clf.predict(X_test)
accuracy = accuracy_score(y_test, y_pred)
print('Accuracy:', accuracy)
```
在上述代码中,我们使用了径向基函数(RBF)作为核函数,并使用粒子群优化算法对支持向量机模型的参数 $C$ 和 $\gamma$ 进行寻优。具体来说,我们将 $C$ 和 $\gamma$ 的取值范围分别设定为 $[10^{-1}, 10^1]$ 和 $[10^{-1}, 10^1]$,并将它们的对数作为粒子位置的维度。在 `update` 方法中,我们使用当前粒子位置构造支持向量机模型,并在训练集上计算分类准确率。最后,我们使用最优参数训练支持向量机模型,并在测试集上测试模型性能。
需要注意的是,这里使用的是径向基函数作为核函数。如果需要使用其他核函数,可以在 `SVC` 的构造函数中设置。另外,由于粒子群优化算法通常是一种全局优化方法,所以在实际应用中需要考虑算法的收敛速度和计算复杂度等问题。
相关推荐
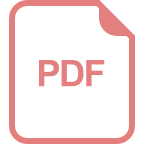













