C语言从json字符串中取出子json字符串,使用json_object.h
时间: 2023-08-17 09:38:31 浏览: 102
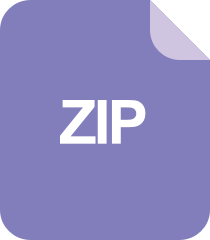
C语言检测字符串是否为json字符串

可以使用第三方库,例如 json-c,来解析 JSON 字符串,然后取出需要的子 JSON 对象。
以下是一个示例代码:
```c
#include <stdio.h>
#include <json-c/json.h>
int main() {
const char *json_str = "{\"person\":{\"name\":\"John\", \"age\":30, \"city\":\"New York\"}}";
json_object *root = json_tokener_parse(json_str);
if (root != NULL) {
json_object *person;
if (json_object_object_get_ex(root, "person", &person)) {
json_object *name;
json_object *age;
json_object *city;
if (json_object_object_get_ex(person, "name", &name)) {
printf("Name: %s\n", json_object_get_string(name));
}
if (json_object_object_get_ex(person, "age", &age)) {
printf("Age: %d\n", json_object_get_int(age));
}
if (json_object_object_get_ex(person, "city", &city)) {
printf("City: %s\n", json_object_get_string(city));
}
}
json_object_put(root);
}
return 0;
}
```
以上代码可以从 JSON 字符串中取出 "person" 子 JSON 对象,然后再从 "person" 中取出 "name"、"age"、"city" 三个子字符串并打印输出。请注意,这里使用 json_tokener_parse 函数将 JSON 字符串解析成 json_object 对象,然后使用 json_object_object_get_ex 函数获取具体的子 JSON 对象和子字符串。最后使用 json_object_put 函数释放对象内存空间。
请注意,使用 json-c 库需要在编译时链接该库,例如使用 gcc 编译时需要加上 `-ljson-c` 参数。
阅读全文
相关推荐

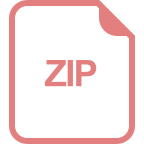
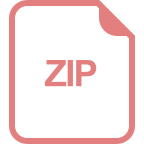
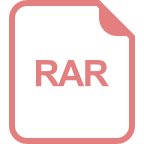
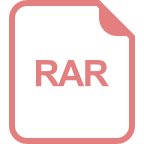
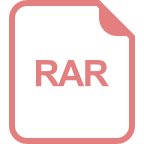
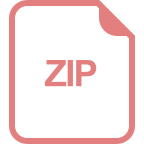
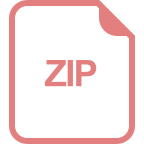
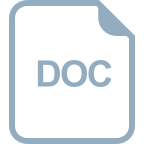







