C语言使用json-c库,打印json字符串
时间: 2023-08-14 07:43:27 浏览: 148
可以使用json-c库中的json_object_to_json_string函数将json_object对象转换为json格式的字符串,然后使用printf函数打印该字符串。示例如下:
```c
#include <stdio.h>
#include <json-c/json.h>
int main() {
// 创建json_object对象
struct json_object *obj = json_object_new_object();
json_object_object_add(obj, "name", json_object_new_string("Alice"));
json_object_object_add(obj, "age", json_object_new_int(20));
// 将json_object对象转换为json格式的字符串
const char *json_str = json_object_to_json_string(obj);
// 打印json字符串
printf("%s\n", json_str);
// 释放json_object对象的内存
json_object_put(obj);
return 0;
}
```
输出结果为:
```
{"name":"Alice","age":20}
```
相关问题
c语言使用json-c
JSON-C是一个C语言库,用于解析、生成和操作JSON数据。它提供了一组简单的API,使开发人员可以轻松地将JSON数据集成到他们的C语言应用程序中。
以下是一个简单的例子,展示了如何使用JSON-C库解析JSON数据:
```c
#include <stdio.h>
#include <json-c/json.h>
int main() {
const char *json_string = "{ \"name\": \"John\", \"age\": 30, \"city\": \"New York\" }";
struct json_object *json_obj = json_tokener_parse(json_string);
// 获取JSON对象中的值
struct json_object *name_obj, *age_obj, *city_obj;
json_object_object_get_ex(json_obj, "name", &name_obj);
json_object_object_get_ex(json_obj, "age", &age_obj);
json_object_object_get_ex(json_obj, "city", &city_obj);
// 打印JSON值
printf("Name: %s\n", json_object_get_string(name_obj));
printf("Age: %d\n", json_object_get_int(age_obj));
printf("City: %s\n", json_object_get_string(city_obj));
// 释放内存
json_object_put(json_obj);
return 0;
}
```
在上面的示例中,我们首先定义了一个JSON字符串,然后使用`json_tokener_parse()`函数将其解析为一个JSON对象。接下来,我们使用`json_object_object_get_ex()`函数获取JSON对象中的各个值。最后,我们使用`json_object_get_string()`和`json_object_get_int()`函数获取字符串和整数值,并将它们打印到控制台上。最后,我们使用`json_object_put()`函数释放JSON对象的内存。
需要注意的是,在使用JSON-C库时,需要在编译时链接`-ljson-c`库。
C语言json-c解析json文件
要解析JSON文件,可以使用json-c库,具体步骤如下:
1. 引入json-c库的头文件
```c
#include <json-c/json.h>
```
2. 读取JSON文件内容
```c
FILE *fp = fopen("filename.json", "r");
if (fp == NULL)
{
printf("Failed to open file\n");
return -1;
}
char buffer[1024];
fread(buffer, 1, 1024, fp);
fclose(fp);
```
3. 解析JSON文件内容
```c
struct json_object *json_obj = json_tokener_parse(buffer);
```
4. 获取JSON对象的值
```c
// 获取字符串类型的值
const char *str_value;
json_object_object_get_ex(json_obj, "key", &str_value);
printf("%s\n", str_value);
// 获取整数类型的值
int int_value;
json_object_object_get_ex(json_obj, "key", &int_value);
printf("%d\n", int_value);
// 获取数组类型的值
struct json_object *arr_obj;
json_object_object_get_ex(json_obj, "key", &arr_obj);
int arr_len = json_object_array_length(arr_obj);
for (int i = 0; i < arr_len; i++)
{
struct json_object *item = json_object_array_get_idx(arr_obj, i);
// 处理数组项
}
```
完整的示例代码如下:
```c
#include <stdio.h>
#include <json-c/json.h>
int main()
{
FILE *fp = fopen("example.json", "r");
if (fp == NULL)
{
printf("Failed to open file\n");
return -1;
}
char buffer[1024];
fread(buffer, 1, 1024, fp);
fclose(fp);
struct json_object *json_obj = json_tokener_parse(buffer);
const char *str_value;
json_object_object_get_ex(json_obj, "name", &str_value);
printf("name: %s\n", str_value);
int age_value;
json_object_object_get_ex(json_obj, "age", &age_value);
printf("age: %d\n", age_value);
struct json_object *hobby_arr_obj;
json_object_object_get_ex(json_obj, "hobbies", &hobby_arr_obj);
int hobby_arr_len = json_object_array_length(hobby_arr_obj);
printf("hobbies:\n");
for (int i = 0; i < hobby_arr_len; i++)
{
struct json_object *item = json_object_array_get_idx(hobby_arr_obj, i);
printf("- %s\n", json_object_get_string(item));
}
json_object_put(json_obj);
return 0;
}
```
需要注意的是,使用完json对象后,需要调用`json_object_put`函数释放内存。
相关推荐
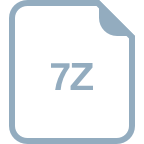
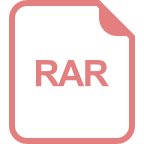












