jave判断字符出现次数
时间: 2023-05-24 21:02:00 浏览: 122
可以使用HashMap来实现统计字符出现次数的功能。具体的实现代码如下:
```java
public static HashMap<Character, Integer> countCharacters(String str) {
HashMap<Character, Integer> map = new HashMap<>();
for (char c : str.toCharArray()) {
map.put(c, map.getOrDefault(c, 0) + 1);
}
return map;
}
// 示例代码
String str = "hello world";
HashMap<Character, Integer> map = countCharacters(str);
for (Map.Entry<Character, Integer> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
```
运行上面的代码,会输出每个字符出现的次数,例如:
```
e: 1
d: 1
h: 1
l: 3
o: 2
r: 1
w: 1
```
注意:上述代码中使用了HashMap类和Map.Entry接口,需要先导入相关的包。
相关问题
java判断字符出现次数
可以通过遍历字符串的每个字符,并使用一个计数器来统计字符出现的次数。
例如,以下是一个简单的例子,用于统计字符串中字符'a'出现的次数:
```java
String str = "Hello World";
char ch = 'a';
int count = 0;
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) == ch) {
count++;
}
}
System.out.println("The character '" + ch + "' appears " + count + " times in the string.");
```
输出结果为:
```
The character 'a' appears 0 times in the string.
```
如果要统计字符串中所有字符出现的次数,可以使用一个Map来保存字符和计数器的对应关系。例如:
```java
String str = "Hello World";
Map<Character, Integer> charCount = new HashMap<>();
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
if (charCount.containsKey(ch)) {
charCount.put(ch, charCount.get(ch) + 1);
} else {
charCount.put(ch, 1);
}
}
System.out.println("Character count: " + charCount);
```
输出结果为:
```
Character count: {H=1, e=1, l=3, o=2, =1, W=1, r=1, d=1}
```
java统计单个字符出现次数
可以使用 HashMap 来存储每个字符出现的次数。具体思路如下:
1. 遍历字符串中的每个字符
2. 判断该字符是否已经在 HashMap 中出现过
- 如果已经出现过,则将对应 value 值加 1
- 如果没有出现过,则将该字符作为 key,value 值设为 1,加入到 HashMap 中
3. 遍历 HashMap,输出每个字符及其出现次数
下面是示例代码:
```java
public static void countChars(String str) {
// 创建 HashMap
HashMap<Character, Integer> map = new HashMap<>();
// 遍历字符串中的每个字符
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
// 判断该字符是否已经在 HashMap 中出现过
if (map.containsKey(c)) {
// 如果已经出现过,则将对应 value 值加 1
int count = map.get(c);
map.put(c, count + 1);
} else {
// 如果没有出现过,则将该字符作为 key,value 值设为 1,加入到 HashMap 中
map.put(c, 1);
}
}
// 遍历 HashMap,输出每个字符及其出现次数
for (Character c : map.keySet()) {
System.out.println(c + ": " + map.get(c));
}
}
```
调用该方法:
```java
String str = "hello world";
countChars(str);
```
输出结果:
```
h: 1
e: 1
l: 3
o: 2
: 1
w: 1
r: 1
d: 1
```
相关推荐
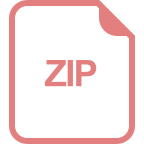












