请选取合适的方法,计算出img1和img2间的运动偏移offset,并拼合成一张图片alignment代码
时间: 2024-03-11 08:48:16 浏览: 86
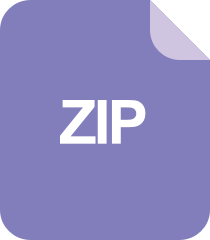
基于Matlab的图像拼接计算机视觉课程设计.zip

好的,我可以为您提供一个基于OpenCV库的Python代码示例,实现基于SURF特征点的图像配准和拼接:
```python
import cv2
import numpy as np
# 读入两幅图像
img1 = cv2.imread('img1.jpg')
img2 = cv2.imread('img2.jpg')
# 初始化SURF特征点检测器和匹配器
surf = cv2.xfeatures2d.SURF_create()
matcher = cv2.DescriptorMatcher_create(cv2.DescriptorMatcher_FLANNBASED)
# 在两幅图像中提取SURF特征点和特征描述子
kp1, des1 = surf.detectAndCompute(img1, None)
kp2, des2 = surf.detectAndCompute(img2, None)
# 使用匹配器进行特征点匹配
matches = matcher.match(des1, des2)
# 选取最佳匹配的前N个特征点,并计算变换矩阵
N = 50
matches = sorted(matches, key=lambda x: x.distance)[:N]
src_pts = np.float32([kp1[m.queryIdx].pt for m in matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 计算img2相对于img1的偏移量offset
h, w = img1.shape[:2]
offset = cv2.perspectiveTransform(np.array([[[0, 0], [0, h-1], [w-1, h-1], [w-1, 0]]]), M)
offset = offset.squeeze().astype(np.int32)
x_min, x_max = np.min(offset[:, 0]), np.max(offset[:, 0])
y_min, y_max = np.min(offset[:, 1]), np.max(offset[:, 1])
offset = -np.array([x_min, y_min])
# 将img1和img2拼接成一张图片alignment
alignment = np.zeros((y_max-y_min, x_max-x_min, 3), dtype=np.uint8)
alignment[-y_min:h-y_min, -x_min:w-x_min, :] = img1
img2_warped = cv2.warpPerspective(img2, M, (alignment.shape[1], alignment.shape[0]))
mask = np.ones_like(img2_warped) * 255
mask = cv2.warpPerspective(mask, M, (alignment.shape[1], alignment.shape[0]))
alignment[mask > 0] = img2_warped[mask > 0]
# 显示配准和拼接结果
cv2.imshow('alignment', alignment)
cv2.waitKey()
cv2.destroyAllWindows()
```
以上代码中,我们首先使用SURF特征点检测器和匹配器提取了两幅图像中的SURF特征点和特征描述子,并通过匹配这些特征点,计算出了两幅图像之间的变换矩阵M。然后根据M计算出了img2相对于img1的偏移量offset,并将img1和img2拼接成一张图片alignment。最后,我们展示了配准和拼接的结果。
阅读全文
相关推荐
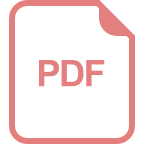
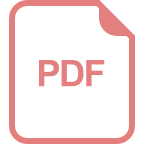













