esp32 dht11
时间: 2023-09-14 21:14:22 浏览: 72
The ESP32 is a powerful microcontroller board that supports WiFi and Bluetooth connectivity. It is widely used in Internet of Things (IoT) applications. The DHT11 is a low-cost temperature and humidity sensor that is commonly used in electronic projects.
When the ESP32 is connected to a DHT11 sensor, it can read the temperature and humidity values from the sensor and transmit them over the WiFi or Bluetooth network. This allows for remote monitoring and control of environmental conditions.
To connect the ESP32 to a DHT11 sensor, you will need to use a library such as the Adafruit DHT library. This library provides functions for reading the temperature and humidity values from the sensor.
Here is an example code for reading temperature and humidity values from a DHT11 sensor using an ESP32:
```
#include <Adafruit_Sensor.h>
#include <DHT.h>
#include <WiFi.h>
#define DHTPIN 2 // pin where the DHT11 sensor is connected
#define DHTTYPE DHT11 // type of the DHT sensor
DHT dht(DHTPIN, DHTTYPE);
const char* ssid = "Your SSID";
const char* password = "Your Password";
void setup() {
Serial.begin(9600);
dht.begin();
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
}
void loop() {
delay(2000); // wait for 2 seconds between readings
float temperature = dht.readTemperature();
float humidity = dht.readHumidity();
Serial.print("Temperature: ");
Serial.print(temperature);
Serial.print(" °C ");
Serial.print("Humidity: ");
Serial.print(humidity);
Serial.println(" %");
}
```
This code reads the temperature and humidity values from the DHT11 sensor and prints them to the serial monitor. It also connects to a WiFi network using the SSID and password provided. You can modify this code to send the temperature and humidity values to a remote server or display them on a web page.
阅读全文
相关推荐
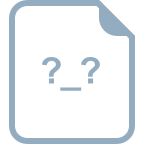








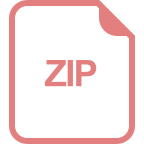
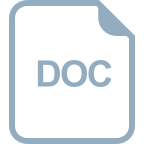





