c#二维数组大量数据采用多线程导出excel,防止异常示例
时间: 2023-11-22 09:27:31 浏览: 108
以下是一个简单的C#代码示例,演示如何使用互斥锁来避免多个线程同时访问Excel文件时出现冲突:
```csharp
using System;
using System.Collections.Generic;
using System.Threading;
using System.Threading.Tasks;
using OfficeOpenXml;
public class ExcelExporter
{
private readonly int threadCount; // 线程数
private readonly int batchSize; // 每个线程处理的数据块大小
private readonly int rowCount; // 数据行数
private readonly int columnCount; // 数据列数
private readonly string[,] data; // 二维数组数据
private readonly string filePath; // Excel文件路径
private readonly object lockObj = new object(); // 互斥锁对象
public ExcelExporter(int threadCount, int batchSize, int rowCount, int columnCount, string[,] data, string filePath)
{
this.threadCount = threadCount;
this.batchSize = batchSize;
this.rowCount = rowCount;
this.columnCount = columnCount;
this.data = data;
this.filePath = filePath;
}
public void Export()
{
using (var package = new ExcelPackage())
{
var worksheet = package.Workbook.Worksheets.Add("Data");
var taskList = new List<Task>();
for (int i = 0; i < threadCount; i++)
{
var startIndex = i * batchSize;
var endIndex = Math.Min(startIndex + batchSize, rowCount);
var task = Task.Factory.StartNew(() =>
{
for (int j = startIndex; j < endIndex; j++)
{
for (int k = 0; k < columnCount; k++)
{
lock (lockObj) // 这里使用互斥锁对象来确保多个线程之间的同步和协调
{
worksheet.Cells[j + 1, k + 1].Value = data[j, k];
}
}
}
});
taskList.Add(task);
}
Task.WaitAll(taskList.ToArray());
package.SaveAs(new System.IO.FileInfo(filePath));
}
}
}
```
在上面的代码中,我们在写入Excel数据的过程中,使用了lock语句来获取互斥锁对象,并在代码块中对Excel数据进行操作,确保多个线程之间的同步和协调,避免出现数据冲突和竞争条件。当一个线程持有互斥锁时,其他线程必须等待该线程释放锁后才能继续执行。这样可以保证多个线程之间互不干扰,避免出现异常情况。
请注意,上面的代码示例仅用于演示如何使用互斥锁来避免多个线程同时访问Excel文件时出现冲突的示例,实际应用中可能需要根据具体情况进行调整和优化。
阅读全文
相关推荐











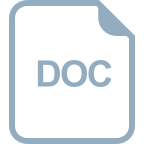






