如何实现redis限流功能
时间: 2023-09-01 08:11:13 浏览: 120
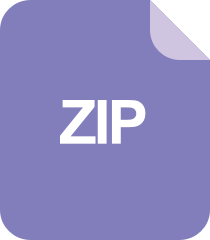
基于redis限流系统

Redis可以通过令牌桶算法、漏桶算法等多种算法实现限流功能,以下是其中一种实现方式:
1. 令牌桶算法实现限流
令牌桶算法是一种基于令牌的限流算法,其原理是在令牌桶中放入一定数量的令牌,每次请求需要从令牌桶中获取令牌才能被处理,当令牌桶中的令牌数量耗尽时,新的请求就会被限流。
实现步骤:
1)设置一个定时器,定时向令牌桶中添加令牌。
2)每次请求需要从令牌桶中获取令牌,如果令牌桶中没有足够的令牌,则限流。
3)实现代码:
```python
import redis
import time
class RedisLimit:
def __init__(self, host, port, password, limit, interval):
self.limit = limit
self.interval = interval
self.redis_conn = redis.StrictRedis(host=host, port=port, password=password, db=0)
def is_limit_exceeded(self, key):
current_time = time.time()
pipeline = self.redis_conn.pipeline()
pipeline.multi()
pipeline.zadd(key, current_time, current_time)
pipeline.zremrangebyscore(key, 0, current_time - self.interval)
pipeline.zcard(key)
count, *_ = pipeline.execute()
return count > self.limit
if __name__ == '__main__':
redis_host = 'localhost'
redis_port = 6379
redis_password = ''
key = 'redis_limit'
limit = 100
interval = 60 # 1分钟内最多访问100次
redis_limit = RedisLimit(redis_host, redis_port, redis_password, limit, interval)
for i in range(200):
if redis_limit.is_limit_exceeded(key):
print('Limit exceeded')
else:
print('Access granted')
```
该代码中使用了Redis的有序集合(sorted set)数据结构来实现令牌桶算法,其中:
- `zadd(key, current_time, current_time)`:向有序集合`key`中添加一个`current_time`的元素,并将其分值(score)设置为`current_time`。
- `zremrangebyscore(key, 0, current_time - self.interval)`:删除有序集合`key`中分值小于等于`current_time - self.interval`的元素。
- `zcard(key)`:返回有序集合`key`的元素个数。
2. 漏桶算法实现限流
漏桶算法是一种基于漏桶的限流算法,其原理是在一个固定容量的漏桶中不断地积累请求,每次请求会从漏桶中流出一定的容量,当漏桶中的容量耗尽时,新的请求就会被限流。
实现步骤:
1)设置一个定时器,定时从漏桶中流出一定的容量。
2)每次请求需要向漏桶中添加请求,如果漏桶已满,则限流。
3)实现代码:
```python
import redis
import time
class RedisLimit:
def __init__(self, host, port, password, limit, interval):
self.limit = limit
self.interval = interval
self.redis_conn = redis.StrictRedis(host=host, port=port, password=password, db=0)
def is_limit_exceeded(self, key):
current_time = time.time()
pipeline = self.redis_conn.pipeline()
pipeline.multi()
pipeline.zadd(key, current_time, current_time)
pipeline.zremrangebyscore(key, 0, current_time - self.interval)
pipeline.zcard(key)
count, *_ = pipeline.execute()
return count > self.limit
if __name__ == '__main__':
redis_host = 'localhost'
redis_port = 6379
redis_password = ''
key = 'redis_limit'
limit = 100
interval = 60 # 1分钟内最多访问100次
redis_limit = RedisLimit(redis_host, redis_port, redis_password, limit, interval)
for i in range(200):
if redis_limit.is_limit_exceeded(key):
print('Limit exceeded')
else:
print('Access granted')
```
该代码中使用了Redis的有序集合(sorted set)数据结构来实现漏桶算法,其中:
- `zadd(key, current_time, current_time)`:向有序集合`key`中添加一个`current_time`的元素,并将其分值(score)设置为`current_time`。
- `zremrangebyscore(key, 0, current_time - self.interval)`:删除有序集合`key`中分值小于等于`current_time - self.interval`的元素。
- `zcard(key)`:返回有序集合`key`的元素个数。
阅读全文
相关推荐
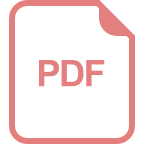
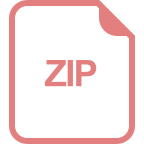















