Spring Cloud Gateway如何实现限流?
时间: 2023-09-14 08:10:25 浏览: 98
Spring Cloud Gateway可以通过集成限流组件来实现限流功能。在Spring Cloud Gateway中,可以使用Redis、Guava、Bucket4j等组件进行限流。
下面以Redis实现限流为例,介绍一种实现方式:
1. 首先,需要引入相关的依赖,例如`spring-boot-starter-data-redis`和`spring-boot-starter-data-redis-reactive`。
2. 在配置文件中配置Redis的连接信息,例如:
```
spring:
redis:
host: localhost
port: 6379
```
3. 创建一个限流过滤器类,实现`GlobalFilter`和`Ordered`接口。例如:
```java
@Component
public class RateLimitFilter implements GlobalFilter, Ordered {
private final RedisTemplate<String, String> redisTemplate;
public RateLimitFilter(RedisTemplate<String, String> redisTemplate) {
this.redisTemplate = redisTemplate;
}
@Override
public Mono<Void> filter(ServerWebExchange exchange, GatewayFilterChain chain) {
String key = exchange.getRequest().getPath().toString();
String limit = redisTemplate.opsForValue().get(key);
if (limit != null && Integer.parseInt(limit) >= 100) {
exchange.getResponse().setStatusCode(HttpStatus.TOO_MANY_REQUESTS);
return exchange.getResponse().setComplete();
}
return chain.filter(exchange);
}
@Override
public int getOrder() {
return -1;
}
}
```
4. 在启动类中注入`RedisTemplate`,并将限流过滤器添加到过滤器链中。例如:
```java
@SpringBootApplication
public class GatewayApplication {
public static void main(String[] args) {
SpringApplication.run(GatewayApplication.class, args);
}
@Autowired
private RedisTemplate<String, String> redisTemplate;
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("rate_limit_route", r -> r.path("/api/**")
.filters(f -> f.filter(new RateLimitFilter(redisTemplate)))
.uri("http://localhost:8080"))
.build();
}
}
```
5. 在Redis中设置限流的阈值。例如,可以使用`redisTemplate.opsForValue().set(key, value)`方法设置限流阈值。
通过以上步骤,就可以在Spring Cloud Gateway中实现基于Redis的限流功能。根据实际需求,可以选择其他限流组件,并进行相应的配置和定制。
相关推荐
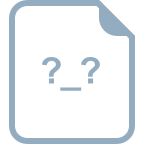
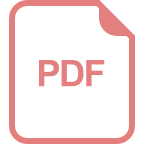













