创建两个线程,要求如下: (1)一个线程输出100个1~26,另一个线程输出100个a~z。 (2)一个线程使用集成Thread类的写法,另一个线程使用实现Runnable接口的写法。
时间: 2024-02-15 10:01:39 浏览: 88
好的,以下是满足你要求的代码:
使用集成Thread类的写法:
```
public class ThreadDemo extends Thread {
private int start;
private int end;
public ThreadDemo(int start, int end) {
this.start = start;
this.end = end;
}
@Override
public void run() {
for (int i = start; i <= end; i++) {
System.out.print((char) (i + 'a' - 1) + " ");
}
}
}
public class Main {
public static void main(String[] args) {
ThreadDemo thread1 = new ThreadDemo(1, 26);
ThreadDemo thread2 = new ThreadDemo(1, 26);
thread1.start();
thread2.start();
}
}
```
使用实现Runnable接口的写法:
```
public class RunnableDemo implements Runnable {
private int start;
private int end;
public RunnableDemo(int start, int end) {
this.start = start;
this.end = end;
}
@Override
public void run() {
for (int i = start; i <= end; i++) {
System.out.print(i + " ");
}
}
}
public class Main {
public static void main(String[] args) {
RunnableDemo runnable1 = new RunnableDemo(1, 26);
RunnableDemo runnable2 = new RunnableDemo(1, 26);
Thread thread1 = new Thread(runnable1);
Thread thread2 = new Thread(runnable2);
thread1.start();
thread2.start();
}
}
```
阅读全文
相关推荐









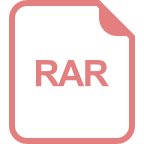



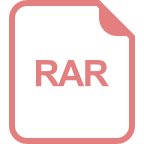



