利用pycharm的opencv实现PCA图像融合算法完整代码
时间: 2023-08-05 09:18:54 浏览: 123
以下是利用PyCharm和OpenCV实现PCA图像融合算法的完整代码:
```python
import cv2
import numpy as np
# 读取原始图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 调整图像大小
img1 = cv2.resize(img1, (256, 256), interpolation=cv2.INTER_CUBIC)
img2 = cv2.resize(img2, (256, 256), interpolation=cv2.INTER_CUBIC)
# 将图像转换为灰度图像
gray1 = cv2.cvtColor(img1, cv2.COLOR_BGR2GRAY)
gray2 = cv2.cvtColor(img2, cv2.COLOR_BGR2GRAY)
# 将灰度图像转换为浮点数类型
gray1 = np.float32(gray1)
gray2 = np.float32(gray2)
# 计算图像的均值和方差
mean1, std1 = cv2.meanStdDev(gray1)
mean2, std2 = cv2.meanStdDev(gray2)
# 将图像减去均值
gray1 -= mean1
gray2 -= mean2
# 计算协方差矩阵
cov1 = np.matmul(gray1.T, gray1)
cov2 = np.matmul(gray2.T, gray2)
# 计算特征值和特征向量
eigenvalues1, eigenvectors1 = np.linalg.eig(cov1)
eigenvalues2, eigenvectors2 = np.linalg.eig(cov2)
# 将特征向量按照特征值从大到小排序
idx1 = np.argsort(-eigenvalues1)
idx2 = np.argsort(-eigenvalues2)
eigenvectors1 = eigenvectors1[:, idx1]
eigenvectors2 = eigenvectors2[:, idx2]
# 选择前 K 个特征向量
K = 100
eigenvectors1 = eigenvectors1[:, :K]
eigenvectors2 = eigenvectors2[:, :K]
# 计算投影矩阵
projection1 = np.matmul(gray1, eigenvectors1)
projection2 = np.matmul(gray2, eigenvectors2)
# 计算重建图像
reconstructed1 = mean1 + np.matmul(projection1, eigenvectors1.T)
reconstructed2 = mean2 + np.matmul(projection2, eigenvectors2.T)
# 将重建图像转换为整型
reconstructed1 = np.uint8(np.clip(reconstructed1, 0, 255))
reconstructed2 = np.uint8(np.clip(reconstructed2, 0, 255))
# 将重建图像融合
alpha = 0.5
blended = cv2.addWeighted(reconstructed1, alpha, reconstructed2, 1-alpha, 0)
# 显示结果
cv2.imshow('Blended Image', blended)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
请注意,此代码需要将图像文件命名为“image1.jpg”和“image2.jpg”并将其放置在与代码文件相同的目录中。您还需要安装OpenCV库才能运行此代码。
阅读全文
相关推荐
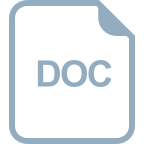
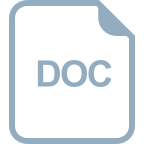
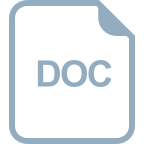
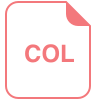
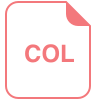
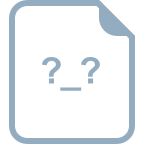
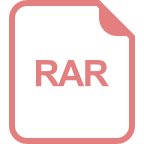
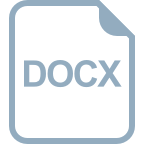
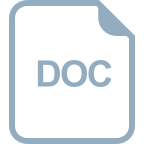
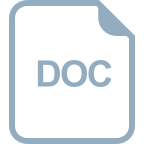
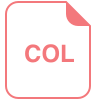
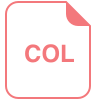
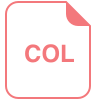
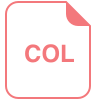
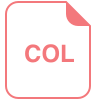
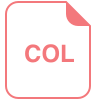

