写一个贪吃蛇的js代码
时间: 2023-05-26 12:04:56 浏览: 41
以下为一个简单的贪吃蛇JavaScript代码,包括一些基本的游戏逻辑和控制:
HTML:
```
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇</title>
<style>
#game-board {
width: 400px;
height: 400px;
border: 1px solid black;
margin: 0 auto;
}
.cell {
width: 20px;
height: 20px;
display: inline-block;
border: 1px solid gray;
box-sizing: border-box;
}
</style>
</head>
<body>
<div id="game-board"></div>
<script src="snake.js"></script>
</body>
</html>
```
JavaScript:
```
var board = document.getElementById("game-board");
var width = 20;
var height = 20;
var score = 0;
var food = { x: 0, y: 0 };
var snake = {
body: [
{ x: 10, y: 10 },
{ x: 9, y: 10 },
{ x: 8, y: 10 }
],
direction: "right"
};
var intervalId = null;
// 初始化游戏面板
function initBoard() {
for (var y = 0; y < height; y++) {
for (var x = 0; x < width; x++) {
var cell = document.createElement("div");
cell.className = "cell";
cell.id = x + "-" + y;
board.appendChild(cell);
}
board.appendChild(document.createElement("br"));
}
}
// 重置游戏
function resetGame() {
score = 0;
snake.body = [
{ x: 10, y: 10 },
{ x: 9, y: 10 },
{ x: 8, y: 10 }
];
snake.direction = "right";
generateFood();
updateScore();
updateSnake();
}
// 更新蛇的位置
function updateSnake() {
// 移动蛇头
var head = snake.body[0];
switch (snake.direction) {
case "right":
head = { x: head.x + 1, y: head.y };
break;
case "left":
head = { x: head.x - 1, y: head.y };
break;
case "up":
head = { x: head.x, y: head.y - 1 };
break;
case "down":
head = { x: head.x, y: head.y + 1 };
break;
}
// 判断是否吃到食物
if (head.x == food.x && head.y == food.y) {
snake.body.unshift(head);
score += 10;
updateScore();
generateFood();
} else {
// 移动蛇尾
var tail = snake.body.pop();
tail.x = head.x;
tail.y = head.y;
snake.body.unshift(tail);
}
// 判断是否撞到墙壁或自己的身体
var headCell = document.getElementById(head.x + "-" + head.y);
if (head.x < 0 || head.x >= width || head.y < 0 || head.y >= height || headCell.className == "cell snake") {
clearInterval(intervalId);
alert("游戏结束,得分:" + score);
resetGame();
} else {
// 更新蛇的位置并重新绘制
updateCells(snake.body, "cell snake");
}
}
// 停止游戏
function stopGame() {
clearInterval(intervalId);
}
// 开始游戏
function startGame() {
resetGame();
intervalId = setInterval(updateSnake, 100);
}
// 更新得分
function updateScore() {
document.getElementById("score").innerHTML = "得分:" + score;
}
// 生成食物
function generateFood() {
var x, y;
do {
x = Math.floor(Math.random() * width);
y = Math.floor(Math.random() * height);
} while (document.getElementById(x + "-" + y).className == "cell snake");
food = { x: x, y: y };
updateCells([food], "cell food");
}
// 更新单元格的类名
function updateCells(cells, className) {
for (var i = 0; i < cells.length; i++) {
var cell = document.getElementById(cells[i].x + "-" + cells[i].y);
cell.className = className || "cell";
}
}
// 键盘事件处理
document.onkeydown = function(e) {
switch (e.keyCode) {
case 37: // left
if (snake.direction != "right") {
snake.direction = "left";
}
break;
case 38: // up
if (snake.direction != "down") {
snake.direction = "up";
}
break;
case 39: // right
if (snake.direction != "left") {
snake.direction = "right";
}
break;
case 40: // down
if (snake.direction != "up") {
snake.direction = "down";
}
break;
}
};
// 启动游戏
initBoard();
startGame();
```
相关推荐
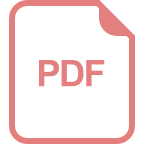





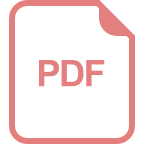
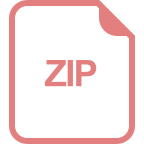
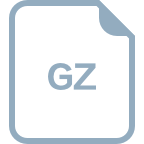
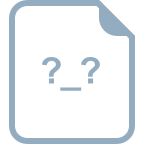