写一个贪吃蛇js代码
时间: 2023-09-19 17:05:05 浏览: 101
### 回答1:
答:
// 初始化
var canvas = document.getElementById('canvas');
var ctx = canvas.getContext('2d');// 创建一个食物
var food = {
x: Math.floor(Math.random() * 20) * 20,
y: Math.floor(Math.random() * 20) * 20
};// 画出贪吃蛇
var snake = {
x: 10 * 20,
y: 10 * 20,
dx: 20,
dy: 0,
cells: [],
maxCells: 2
};// 把贪吃蛇的部分放进数组
for(var i = 0; i < snake.maxCells; i++) {
snake.cells.push({x: snake.x, y: snake.y});
}// 更新贪吃蛇
function update() {
// 把蛇头往前走一步
snake.x += snake.dx;
snake.y += snake.dy; // 碰到墙壁就反弹
if(snake.x < 0) {
snake.x = canvas.width - snake.dx;
}
if(snake.x >= canvas.width) {
snake.x = 0;
}
if(snake.y < 0) {
snake.y = canvas.height - snake.dy;
}
if(snake.y >= canvas.height) {
snake.y = 0;
} // 把蛇头前进的一步加到数组
snake.cells.unshift({x: snake.x, y: snake.y}); // 如果蛇的长度大于最大长度,则删除最后一个
if(snake.cells.length > snake.maxCells) {
snake.cells.pop();
} // 判断蛇头是否碰到食物
if(snake.x == food.x && snake.y == food.y) {
// 增加蛇的长度
snake.maxCells++; // 食物重新出现
food.x = Math.floor(Math.random() * 20) * 20;
food.y = Math.floor(Math.random() * 20) * 20;
}
}// 把贪吃蛇画出来
function draw() {
// 把画布清空
ctx.clearRect(0, 0, canvas.width, canvas.height); // 画出贪吃蛇
ctx.fillStyle = '#00FF00';
snake.cells.forEach(function(cell, index) {
ctx.fillRect(cell.x, cell.y, 20, 20); // 蛇头
if(index == 0) {
ctx.fillStyle = '#FF0000';
ctx.fillRect(cell.x, cell.y, 20, 20);
}
}); // 画出食物
ctx.fillStyle = '#FF0000';
ctx.fillRect(food.x, food.y, 20, 20);
}// 把更新和画出来放进循环
function loop() {
update();
draw();
requestAnimationFrame(loop);
}// 启动游戏
requestAnimationFrame(loop);
### 回答2:
贪吃蛇是一款经典的游戏,下面是一个简单的贪吃蛇的Javascript代码示例,实现了基本的游戏功能:
HTML部分:
```
<!DOCTYPE html>
<html>
<head>
<title>贪吃蛇游戏</title>
<style>
#game-board {
width: 600px;
height: 400px;
margin: 0 auto;
border: 1px solid black;
position: relative;
}
.snake {
width: 20px;
height: 20px;
position: absolute;
background-color: green;
}
.food {
width: 20px;
height: 20px;
position: absolute;
background-color: red;
}
</style>
</head>
<body>
<div id="game-board"></div>
<script src="snake.js"></script>
</body>
</html>
```
Javascript部分(snake.js):
```
// 获取游戏区域和初始化蛇身
const gameBoard = document.getElementById("game-board");
const snake = [{x: 10, y: 10}];
let direction = {x: 0, y: 0};
let food = {x: 0, y: 0};
// 生成随机食物
function generateFood() {
food.x = Math.floor(Math.random() * 30) * 20;
food.y = Math.floor(Math.random() * 20) * 20;
}
// 绘制游戏区域和蛇身
function drawGameBoard() {
gameBoard.innerHTML = "";
snake.forEach(segment => {
const snakeSegment = document.createElement("div");
snakeSegment.style.left = segment.x + "px";
snakeSegment.style.top = segment.y + "px";
snakeSegment.classList.add("snake");
gameBoard.appendChild(snakeSegment);
});
const foodElement = document.createElement("div");
foodElement.style.left = food.x + "px";
foodElement.style.top = food.y + "px";
foodElement.classList.add("food");
gameBoard.appendChild(foodElement);
}
// 移动蛇身
function moveSnake() {
const head = {...snake[0]};
snake.unshift({x: head.x + direction.x, y: head.y + direction.y});
snake.pop();
}
// 监听键盘事件
document.addEventListener("keydown", e => {
if (e.key === "ArrowUp" && direction.y !== 1) {
direction = {x: 0, y: -1};
} else if (e.key === "ArrowDown" && direction.y !== -1) {
direction = {x: 0, y: 1};
} else if (e.key === "ArrowLeft" && direction.x !== 1) {
direction = {x: -1, y: 0};
} else if (e.key === "ArrowRight" && direction.x !== -1) {
direction = {x: 1, y: 0};
}
});
// 主循环
function gameLoop() {
moveSnake();
if (snake[0].x === food.x && snake[0].y === food.y) {
generateFood();
snake.push({});
}
drawGameBoard();
setTimeout(gameLoop, 100);
}
// 启动游戏
generateFood();
gameLoop();
```
这是一个简化版本的贪吃蛇游戏,其中包含基本的移动、吃食物和撞墙判定等功能。你可以通过上下左右键来控制贪吃蛇的移动方向,在游戏区域内吃食物增加蛇的长度,当蛇撞到墙壁或者自己的身体时游戏结束。
### 回答3:
贪吃蛇是经典的游戏之一,现在我将用300字来编写一个简单的贪吃蛇JS代码。
首先,我们需要定义蛇的初始位置和初始方向。我们可以使用一个数组来表示蛇的身体,其中每个元素代表一个蛇节的位置。初始时,蛇身体的长度为3,位置坐标为(0, 0),方向向右。
接下来,我们需要定义食物的位置,食物的位置是随机生成的,当蛇吃到食物时,蛇的长度会增加1,同时生成一个新的食物位置。
在游戏的主循环中,我们需要监听玩家的按键操作,当玩家按下上、下、左、右键时,我们需要改变蛇的方向。蛇的移动是根据蛇头的位置和方向来确定的,每个蛇节都会跟随前一个蛇节进行移动。
在每一次循环中,我们需要检测蛇头是否碰到了蛇身体或者墙壁,如果是,则游戏结束。同时,我们还需要检测蛇头是否碰到了食物,如果是,则蛇的长度增加1,生成一个新的食物位置。
最后,我们需要绘制游戏界面。可以使用HTML5的canvas元素来实现绘制,通过绘制矩形来表示蛇的身体和食物,每一帧都要清空画布并重新绘制。
以上就是一个简单的贪吃蛇JS代码的实现思路,具体的代码可以根据需求进行扩展和改进。希望对你有帮助!
阅读全文
相关推荐





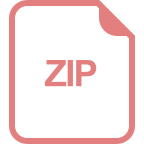
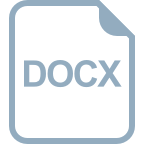