class MainWindow(QMainWindow): def init(self): super().init() self.setFixedSize(800, 600) main_layout = QVBoxLayout() central_widget = QWidget() central_widget.setLayout(main_layout) self.setCentralWidget(central_widget) button_layout = QVBoxLayout() button1 = QPushButton('当日员工工资') button1.setFixedSize(200, 50) button1.clicked.connect(self.show_query1_result) button_layout.addStretch() button_layout.addWidget(button1) button_layout.addStretch() layout = QHBoxLayout() layout.addStretch() layout.addLayout(button_layout) layout.addStretch() widget = QWidget() widget.setLayout(layout) self.setCentralWidget(widget) main_layout.addLayout(button_layout) self.query1_window = QueryResultWindow() def show_query1_result(self): db = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', db='jj_tset') cursor = db.cursor() db_sql = """ """ cursor.execute(db_sql) result = cursor.fetchall() db.close() if len(result) == 0: QMessageBox.information(self, "提示", "今日无员工工资记录") return self.query1_window.table_widget.setRowCount(0) self.query1_window.table_widget.setColumnCount(len(result[0])) self.query1_window.table_widget.setHorizontalHeaderLabels( ["员工ID", "员工姓名", "日期", "领取鸡爪重量(KG)", "效率(每小时KG)", "出成率", "基础工资", "重量奖励", "当日总工资"]) for row_num, row_data in enumerate(result): self.query1_window.table_widget.insertRow(row_num) for col_num, col_data in enumerate(row_data): self.query1_window.table_widget.setItem(row_num, col_num, QTableWidgetItem(str(col_data))) self.query1_window.show() class QueryResultWindow(QWidget): def init(self): super().init() self.setFixedSize(800, 600) self.table_widget = QTableWidget() self.table_widget.setEditTriggers(QTableWidget.NoEditTriggers) self.table_widget.setSelectionBehavior(QTableWidget.SelectRows) layout = QVBoxLayout() layout.addWidget(self.table_widget) self.setLayout(layout) if name == 'main': app = QApplication(sys.argv) loginWindow = LoginWindow() loginWindow.show() sys.exit(app.exec_()))数据展示页面怎么设置筛选器按ID筛选结果并展示的整体代码
时间: 2023-08-02 16:09:03 浏览: 161
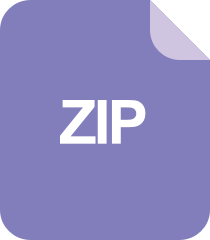
QT_UART.zip
以下是整体代码:
```
import sys
import pymysql
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QVBoxLayout, QHBoxLayout, QPushButton, QMessageBox, QTableWidget, QTableWidgetItem
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.setFixedSize(800, 600)
main_layout = QVBoxLayout()
central_widget = QWidget()
central_widget.setLayout(main_layout)
self.setCentralWidget(central_widget)
button_layout = QVBoxLayout()
button1 = QPushButton('当日员工工资')
button1.setFixedSize(200, 50)
button1.clicked.connect(self.show_query1_result)
button_layout.addStretch()
button_layout.addWidget(button1)
button_layout.addStretch()
layout = QHBoxLayout()
layout.addStretch()
layout.addLayout(button_layout)
layout.addStretch()
widget = QWidget()
widget.setLayout(layout)
self.setCentralWidget(widget)
main_layout.addLayout(button_layout)
self.query1_window = QueryResultWindow()
def show_query1_result(self):
db = pymysql.connect(host='39.99.214.172', user='root', password='Solotion.123', db='jj_tset')
cursor = db.cursor()
db_sql = """SELECT * FROM employee_salary WHERE emp_id = %s"""
emp_id, okPressed = QInputDialog.getInt(self, "员工ID筛选", "请输入员工ID:", 0, 0, 999999, 1)
if not okPressed:
return
cursor.execute(db_sql, (emp_id,))
result = cursor.fetchall()
db.close()
if len(result) == 0:
QMessageBox.information(self, "提示", "今日无员工工资记录")
return
self.query1_window.table_widget.setRowCount(0)
self.query1_window.table_widget.setColumnCount(len(result[0]))
self.query1_window.table_widget.setHorizontalHeaderLabels(["员工ID", "员工姓名", "日期", "领取鸡爪重量(KG)", "效率(每小时KG)", "出成率", "基础工资", "重量奖励", "当日总工资"])
for row_num, row_data in enumerate(result):
self.query1_window.table_widget.insertRow(row_num)
for col_num, col_data in enumerate(row_data):
self.query1_window.table_widget.setItem(row_num, col_num, QTableWidgetItem(str(col_data)))
self.query1_window.show()
class QueryResultWindow(QWidget):
def __init__(self):
super().__init__()
self.setFixedSize(800, 600)
self.table_widget = QTableWidget()
self.table_widget.setEditTriggers(QTableWidget.NoEditTriggers)
self.table_widget.setSelectionBehavior(QTableWidget.SelectRows)
layout = QVBoxLayout()
layout.addWidget(self.table_widget)
self.setLayout(layout)
if __name__ == '__main__':
app = QApplication(sys.argv)
mainWindow = MainWindow()
mainWindow.show()
sys.exit(app.exec_())
```
主要是在 `show_query1_result` 方法中添加了一个输入框,用于输入要筛选的员工ID,然后根据输入的ID从数据库中查询数据并展示在表格中。注意要导入 `QInputDialog`,这是一个用于获取用户输入的对话框。
阅读全文