使用Java语言实现一个贪吃蛇小游戏
时间: 2023-06-18 14:04:24 浏览: 114
好的,下面是一个简单的贪吃蛇小游戏的Java代码:
```
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class SnakeGame extends JFrame implements ActionListener {
private static final long serialVersionUID = -4272056262246670918L;
private JPanel gamePanel;
private JButton startButton, stopButton;
private Timer timer;
private int delay = 200; // 蛇移动的速度
private int score = 0; // 得分
private int snakeLength = 3; // 蛇的长度
private int[] snakeX = new int[100]; // 蛇身体的X坐标
private int[] snakeY = new int[100]; // 蛇身体的Y坐标
private int foodX; // 食物的X坐标
private int foodY; // 食物的Y坐标
private int direction = 1; // 蛇的方向,1表示向上,2表示向下,3表示向左,4表示向右
public SnakeGame() {
super("贪吃蛇小游戏");
gamePanel = new JPanel();
gamePanel.setLayout(null);
gamePanel.setPreferredSize(new Dimension(400, 400));
gamePanel.setBackground(Color.WHITE);
getContentPane().add(gamePanel, BorderLayout.CENTER);
startButton = new JButton("开始游戏");
startButton.setBounds(50, 420, 100, 30);
startButton.addActionListener(this);
gamePanel.add(startButton);
stopButton = new JButton("结束游戏");
stopButton.setBounds(250, 420, 100, 30);
stopButton.addActionListener(this);
gamePanel.add(stopButton);
timer = new Timer(delay, this);
initGame();
}
private void initGame() {
score = 0;
snakeLength = 3;
snakeX[0] = 100;
snakeY[0] = 100;
snakeX[1] = 75;
snakeY[1] = 100;
snakeX[2] = 50;
snakeY[2] = 100;
foodX = (int) (Math.random() * 380 / 10) * 10;
foodY = (int) (Math.random() * 380 / 10) * 10;
direction = 1;
}
private void paintSnake(Graphics g) {
for (int i = 0; i < snakeLength; i++) {
if (i == 0) {
g.setColor(Color.RED);
} else {
g.setColor(Color.BLACK);
}
g.fillRect(snakeX[i], snakeY[i], 10, 10);
}
}
private void paintFood(Graphics g) {
g.setColor(Color.GREEN);
g.fillOval(foodX, foodY, 10, 10);
}
private boolean isEatFood() {
if (snakeX[0] == foodX && snakeY[0] == foodY) {
return true;
} else {
return false;
}
}
private boolean isGameOver() {
if (snakeX[0] < 0 || snakeX[0] > 390 || snakeY[0] < 0 || snakeY[0] > 390) {
return true;
}
for (int i = 1; i < snakeLength; i++) {
if (snakeX[i] == snakeX[0] && snakeY[i] == snakeY[0]) {
return true;
}
}
return false;
}
private void moveSnake() {
for (int i = snakeLength - 1; i > 0; i--) {
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
if (direction == 1) {
snakeY[0] -= 10;
} else if (direction == 2) {
snakeY[0] += 10;
} else if (direction == 3) {
snakeX[0] -= 10;
} else if (direction == 4) {
snakeX[0] += 10;
}
}
private void createNewFood() {
foodX = (int) (Math.random() * 380 / 10) * 10;
foodY = (int) (Math.random() * 380 / 10) * 10;
for (int i = 0; i < snakeLength; i++) {
if (snakeX[i] == foodX && snakeY[i] == foodY) {
createNewFood();
break;
}
}
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == startButton) {
initGame();
timer.start();
gamePanel.requestFocus();
} else if (e.getSource() == stopButton) {
timer.stop();
} else if (e.getSource() == timer) {
moveSnake();
if (isGameOver()) {
timer.stop();
JOptionPane.showMessageDialog(gamePanel, "游戏结束,得分为:" + score, "游戏结束", JOptionPane.INFORMATION_MESSAGE);
} else if (isEatFood()) {
score += 10;
snakeLength++;
createNewFood();
}
gamePanel.repaint();
}
}
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_UP && direction != 2) {
direction = 1;
} else if (e.getKeyCode() == KeyEvent.VK_DOWN && direction != 1) {
direction = 2;
} else if (e.getKeyCode() == KeyEvent.VK_LEFT && direction != 4) {
direction = 3;
} else if (e.getKeyCode() == KeyEvent.VK_RIGHT && direction != 3) {
direction = 4;
}
}
public static void main(String[] args) {
SnakeGame snakeGame = new SnakeGame();
snakeGame.setSize(400, 500);
snakeGame.setResizable(false);
snakeGame.setLocationRelativeTo(null);
snakeGame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
snakeGame.setVisible(true);
}
}
```
这个代码使用了Java Swing界面库来实现界面,使用了一个定时器来控制蛇的移动,使用了键盘事件来控制蛇的方向。在界面上有开始游戏和结束游戏两个按钮,还有得分的显示。在游戏开始时,初始化蛇的位置、长度、方向和食物的位置。在定时器的每次触发中,先判断游戏是否结束,然后再判断蛇是否吃到了食物,如果吃到了就增加得分和蛇的长度,并重新生成食物。最后更新界面,重绘蛇和食物。
阅读全文
相关推荐
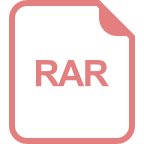

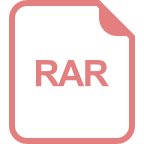


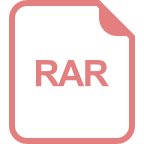







