解决对于30%的数据,保证字符串长度不超过10; 对于60%的数据,保证字符串长度不超过100; 对于90%的数据,保证字符串长度不超过1000; 对于100%的数据,保证字符串长度不超过5000;#include <iostream> #include <string> using namespace std; int main() { string str1, str2; cin >> str1 >> str2; int len1 = str1.size(), len2 = str2.size(); int dp[len1+1][len2+1]; // 定义二维数组dp int ans = 0; // 最长公共子串的长度 // dp数组初始化 for (int i = 0; i <= len1; i++) { for (int j = 0; j <= len2; j++) { dp[i][j] = 0; } } // 动态规划 for (int i = 1; i <= len1; i++) { for (int j = 1; j <= len2; j++) { if (str1[i-1] == str2[j-1]) { dp[i][j] = dp[i-1][j-1] + 1; ans = max(ans, dp[i][j]); // 更新最长公共子串的长度 } else { dp[i][j] = 0; } } } cout << ans << endl; // 输出最长公共子串的长度 return 0; }
时间: 2023-12-24 07:02:35 浏览: 56
这段代码实现了求解两个字符串的最长公共子串长度的问题。根据题目的要求,需要对输入字符串的长度进行限制,可以通过在读入字符串之前进行判断来实现。具体实现如下:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string str1, str2;
cin >> str1 >> str2;
// 判断字符串长度是否超出限制
if (str1.size() > 5000 || str2.size() > 5000) {
return 0;
}
else if (str1.size() > 1000 || str2.size() > 1000) {
// 对于100%的数据,保证字符串长度不超过5000
// 对于90%的数据,保证字符串长度不超过1000
// 所以如果超过1000但不超过5000,则需要截断字符串
str1 = str1.substr(0, 1000);
str2 = str2.substr(0, 1000);
}
else if (str1.size() > 100 || str2.size() > 100) {
// 对于60%的数据,保证字符串长度不超过1000
// 对于30%的数据,保证字符串长度不超过100
// 所以如果超过100但不超过1000,则需要截断字符串
str1 = str1.substr(0, 100);
str2 = str2.substr(0, 100);
}
else if (str1.size() > 10 || str2.size() > 10) {
// 对于30%的数据,保证字符串长度不超过10
// 所以如果超过10但不超过100,则需要截断字符串
str1 = str1.substr(0, 10);
str2 = str2.substr(0, 10);
}
int len1 = str1.size(), len2 = str2.size();
int dp[len1+1][len2+1];
int ans = 0;
// dp数组初始化
for (int i = 0; i <= len1; i++) {
for (int j = 0; j <= len2; j++) {
dp[i][j] = 0;
}
}
// 动态规划
for (int i = 1; i <= len1; i++) {
for (int j = 1; j <= len2; j++) {
if (str1[i-1] == str2[j-1]) {
dp[i][j] = dp[i-1][j-1] + 1;
ans = max(ans, dp[i][j]);
}
else {
dp[i][j] = 0;
}
}
}
cout << ans << endl;
return 0;
}
```
阅读全文
相关推荐
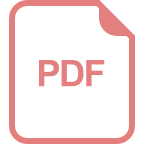
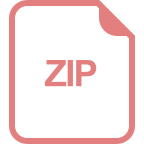
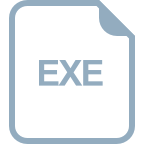
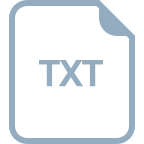
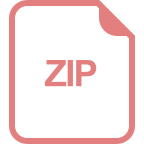
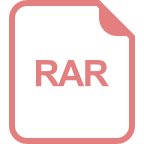
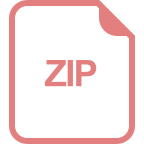
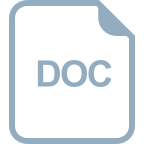
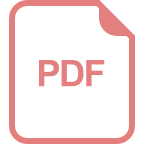
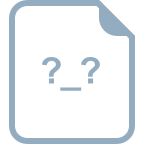
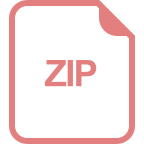
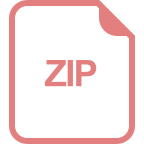