android studio播放器页面
时间: 2023-09-14 21:10:09 浏览: 61
要创建一个播放器页面,您可以使用Android Studio中的MediaPlayer API和XML布局来构建。以下是一个简单的示例:
1. 首先,您需要在XML布局文件中定义一个MediaPlayer控件和一些控件按钮,如播放、暂停、停止和进度条等等。
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<VideoView
android:id="@+id/videoView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_centerInParent="true"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:paddingTop="10dp"
android:paddingBottom="10dp"
android:paddingLeft="16dp"
android:paddingRight="16dp">
<Button
android:id="@+id/buttonPlay"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Play"/>
<Button
android:id="@+id/buttonPause"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Pause"/>
<Button
android:id="@+id/buttonStop"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Stop"/>
<SeekBar
android:id="@+id/seekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"/>
</LinearLayout>
</RelativeLayout>
```
2. 接下来,在您的Java代码中,您需要实例化MediaPlayer和控件按钮,并设置它们的监听器。您需要编写代码来处理按钮的单击事件和SeekBar的滑动事件。
```
public class MainActivity extends AppCompatActivity {
private VideoView videoView;
private Button buttonPlay;
private Button buttonPause;
private Button buttonStop;
private SeekBar seekBar;
private MediaPlayer mediaPlayer;
private int totalTime;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
videoView = findViewById(R.id.videoView);
buttonPlay = findViewById(R.id.buttonPlay);
buttonPause = findViewById(R.id.buttonPause);
buttonStop = findViewById(R.id.buttonStop);
seekBar = findViewById(R.id.seekBar);
mediaPlayer = MediaPlayer.create(this, R.raw.sample_video);
mediaPlayer.setLooping(true);
mediaPlayer.seekTo(0);
totalTime = mediaPlayer.getDuration();
seekBar.setMax(totalTime);
buttonPlay.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (!mediaPlayer.isPlaying()) {
mediaPlayer.start();
}
}
});
buttonPause.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mediaPlayer.isPlaying()) {
mediaPlayer.pause();
}
}
});
buttonStop.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (mediaPlayer.isPlaying()) {
mediaPlayer.stop();
mediaPlayer.seekTo(0);
}
}
});
seekBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
if (fromUser) {
mediaPlayer.seekTo(progress);
seekBar.setProgress(progress);
}
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
}
});
new Thread(new Runnable() {
@Override
public void run() {
while (mediaPlayer != null) {
try {
Message message = new Message();
message.what = mediaPlayer.getCurrentPosition();
handler.sendMessage(message);
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}).start();
}
private Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
int currentPosition = msg.what;
seekBar.setProgress(currentPosition);
String elapsedTime = createTimeLabel(currentPosition);
String remainingTime = createTimeLabel(totalTime - currentPosition);
((TextView) findViewById(R.id.textElapsedTime)).setText(elapsedTime);
((TextView) findViewById(R.id.textRemainingTime)).setText("-" + remainingTime);
}
};
public String createTimeLabel(int time) {
String timeLabel = "";
int min = time / 1000 / 60;
int sec = time / 1000 % 60;
timeLabel = min + ":";
if (sec < 10) timeLabel += "0";
timeLabel += sec;
return timeLabel;
}
@Override
protected void onDestroy() {
super.onDestroy();
mediaPlayer.release();
mediaPlayer = null;
}
}
```
3. 最后,您需要准备一些示例视频文件,并将其放入您的Android项目的“raw”文件夹中。在这个示例应用程序中,我们使用一个名为“sample_video.mp4”的视频文件,并将其放入“res/raw”文件夹中。
这就是创建一个播放器页面的基本过程。您可以根据需要添加更多的功能和控件。
相关推荐
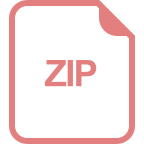
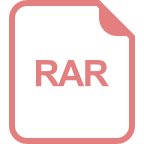
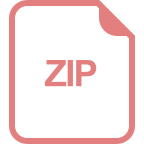













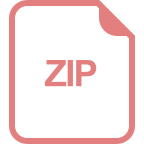
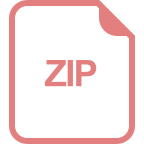