; 配置文件 [section1] key=工作路径 value=C:/Users/lenovo/Desktop [section2] key=壳体模型路径 value=C:/Users/lenovo/Desktop [section3] key=网格尺寸 value=4 [section4] key=壳体内壁压强 value=0.1 使用MFC 实现ini文件的读写操作
时间: 2024-02-05 15:13:05 浏览: 71
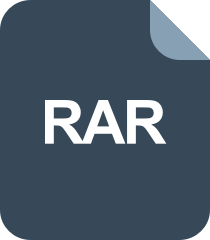
MFC读写ini配置文件

下面是一个使用 MFC 实现 ini 文件读写的示例代码:
```c++
#include <afxwin.h>
#include <afxext.h> // 必须包含此头文件
// 读取 ini 文件
void ReadIniFile()
{
CString strFilePath = _T("config.ini"); // ini 文件路径
CString strSection1 = _T("section1");
CString strSection2 = _T("section2");
CString strSection3 = _T("section3");
CString strSection4 = _T("section4");
CString strKey1 = _T("key");
CString strKey2 = _T("key");
CString strKey3 = _T("key");
CString strKey4 = _T("key");
CString strValue1;
CString strValue2;
CString strValue3;
CString strValue4;
strValue1.GetBuffer(MAX_PATH);
strValue2.GetBuffer(MAX_PATH);
strValue3.GetBuffer(MAX_PATH);
strValue4.GetBuffer(MAX_PATH);
// 读取 section1 中的 key 值
GetPrivateProfileString(strSection1, strKey1, _T(""), strValue1.GetBuffer(MAX_PATH), MAX_PATH, strFilePath);
// 读取 section2 中的 key 值
GetPrivateProfileString(strSection2, strKey2, _T(""), strValue2.GetBuffer(MAX_PATH), MAX_PATH, strFilePath);
// 读取 section3 中的 key 值
GetPrivateProfileString(strSection3, strKey3, _T(""), strValue3.GetBuffer(MAX_PATH), MAX_PATH, strFilePath);
// 读取 section4 中的 key 值
GetPrivateProfileString(strSection4, strKey4, _T(""), strValue4.GetBuffer(MAX_PATH), MAX_PATH, strFilePath);
// 打印读取到的值
TRACE(_T("section1->key: %s\n"), strValue1);
TRACE(_T("section2->key: %s\n"), strValue2);
TRACE(_T("section3->key: %s\n"), strValue3);
TRACE(_T("section4->key: %s\n"), strValue4);
}
// 写入 ini 文件
void WriteIniFile()
{
CString strFilePath = _T("config.ini"); // ini 文件路径
CString strSection1 = _T("section1");
CString strSection2 = _T("section2");
CString strSection3 = _T("section3");
CString strSection4 = _T("section4");
CString strKey1 = _T("key");
CString strKey2 = _T("key");
CString strKey3 = _T("key");
CString strKey4 = _T("key");
CString strValue1 = _T("C:/Users/lenovo/Desktop");
CString strValue2 = _T("C:/Users/lenovo/Desktop");
CString strValue3 = _T("4");
CString strValue4 = _T("0.1");
// 写入 section1 中的 key 值
WritePrivateProfileString(strSection1, strKey1, strValue1, strFilePath);
// 写入 section2 中的 key 值
WritePrivateProfileString(strSection2, strKey2, strValue2, strFilePath);
// 写入 section3 中的 key 值
WritePrivateProfileString(strSection3, strKey3, strValue3, strFilePath);
// 写入 section4 中的 key 值
WritePrivateProfileString(strSection4, strKey4, strValue4, strFilePath);
}
int main()
{
// 写入 ini 文件
WriteIniFile();
// 读取 ini 文件
ReadIniFile();
return 0;
}
```
该示例代码中使用了 MFC 提供的函数 `GetPrivateProfileString` 和 `WritePrivateProfileString` 来读取和写入 ini 文件。其中,`GetPrivateProfileString` 函数用于读取 ini 文件中指定的 section 和 key 对应的值,而 `WritePrivateProfileString` 函数用于将指定的值写入 ini 文件中的 section 和 key 中。
阅读全文
相关推荐
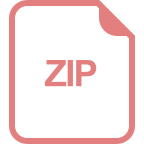




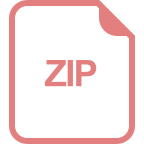
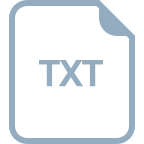
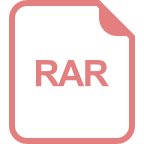
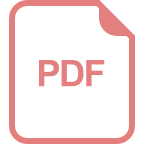





