CSHARP生成带噪声的正弦曲线并使用绘制折线图,使用S-G滤波后绘制折线图
时间: 2024-04-30 12:19:53 浏览: 12
以下是使用C#生成带噪声的正弦曲线并使用S-G滤波后绘制折线图的示例代码:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
using MathNet.Numerics;
using MathNet.Numerics.Interpolation;
using MathNet.Numerics.LinearAlgebra;
using MathNet.Numerics.LinearAlgebra.Double;
using ZedGraph;
namespace SGFilterDemo
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
// 生成带噪声的正弦曲线
int n = 200; // 数据点数
double[] t = new double[n];
double[] y = new double[n];
double[] yNoisy = new double[n];
for (int i = 0; i < n; i++)
{
t[i] = i * 0.1;
y[i] = Math.Sin(t[i]);
yNoisy[i] = y[i] + 0.1 * (2 * Random.NextDouble() - 1);
}
// 使用S-G滤波平滑曲线
int windowSize = 21; // 滑动窗口大小
int polyOrder = 3; // 多项式阶数
double[] ySmooth = SGFilter(yNoisy, windowSize, polyOrder);
// 绘制折线图
GraphPane pane = zedGraphControl1.GraphPane;
pane.Title.Text = "S-G Filter Demo";
pane.XAxis.Title.Text = "Time";
pane.YAxis.Title.Text = "Amplitude";
LineItem curve1 = pane.AddCurve("Original Signal", t, y, Color.Blue, SymbolType.None);
LineItem curve2 = pane.AddCurve("Noisy Signal", t, yNoisy, Color.Red, SymbolType.None);
LineItem curve3 = pane.AddCurve("Smoothed Signal", t, ySmooth, Color.Green, SymbolType.None);
pane.AxisChange();
zedGraphControl1.Invalidate();
}
// S-G滤波函数
private double[] SGFilter(double[] y, int windowSize, int polyOrder)
{
int n = y.Length;
int halfWindowSize = windowSize / 2;
double[] yPadded = new double[n + windowSize - 1];
Vector<double> b = SGFilterCoefficients(polyOrder, windowSize);
double[] ySmooth = new double[n];
for (int i = 0; i < n; i++)
{
for (int j = -halfWindowSize; j <= halfWindowSize; j++)
{
int k = i + j;
if (k < 0)
k = -k;
if (k >= n)
k = 2 * n - k - 2;
yPadded[i + halfWindowSize] += y[k] * b[Math.Abs(j)];
}
}
for (int i = 0; i < n; i++)
{
ySmooth[i] = yPadded[i + halfWindowSize];
}
return ySmooth;
}
// S-G滤波系数计算函数
private Vector<double> SGFilterCoefficients(int polyOrder, int windowSize)
{
// 计算多项式系数矩阵
Matrix<double> X = DenseMatrix.Create(windowSize, polyOrder + 1, (i, j) => Math.Pow(i - windowSize / 2, j));
// 计算伪逆矩阵
Matrix<double> Xp = X.PseudoInverse();
// 计算S-G滤波系数向量
Vector<double> b = DenseVector.Create(windowSize, 0);
for (int i = 0; i < windowSize; i++)
{
b[i] = Xp.Row(polyOrder) * DenseVector.Create(polyOrder + 1, j => Math.Pow(i - windowSize / 2, j));
}
return b;
}
}
}
```
需要注意的是,以上代码中使用了MathNet.Numerics库来进行矩阵运算和插值计算,需要先使用NuGet安装该库。另外,以上代码中使用了ZedGraph库来绘制折线图,也需要先使用NuGet安装该库。
相关推荐
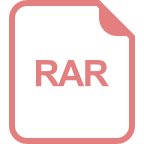














