CSHARP生成带噪声的正弦曲线并使用drawing绘制折线图,使用S-G滤波后绘制折线图
时间: 2024-05-11 14:20:09 浏览: 131
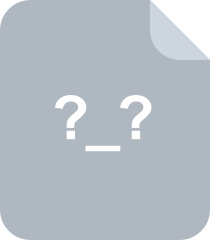
C语言程序绘制正弦曲线
以下是在C#中生成带噪声的正弦曲线并使用Drawing绘制折线图,然后使用S-G滤波后绘制折线图的示例代码:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
namespace SinWaveWithNoise
{
public partial class Form1 : Form
{
private const int Width = 600;
private const int Height = 400;
private const int Margin = 50;
private const int NumPoints = 100;
private readonly Random _random = new Random();
private readonly PointF[] _points = new PointF[NumPoints];
private readonly PointF[] _filteredPoints = new PointF[NumPoints];
private readonly Pen _sinPen = new Pen(Color.Red, 2f);
private readonly Pen _noisePen = new Pen(Color.Blue, 1f);
private readonly Pen _filteredPen = new Pen(Color.Green, 2f);
public Form1()
{
InitializeComponent();
// Generate noisy sine wave points
for (int i = 0; i < NumPoints; i++)
{
float x = (float) i / (NumPoints - 1) * (Width - 2 * Margin) + Margin;
float y = (float) (Math.Sin(x * 2 * Math.PI / (Width - 2 * Margin)) + 0.5 * _random.NextDouble() - 0.25);
_points[i] = new PointF(x, y * (Height - 2 * Margin) + Margin);
}
// Apply S-G filter
float[] coefficients = { -3f / 35f, 12f / 35f, 17f / 35f, 12f / 35f, -3f / 35f };
for (int i = 2; i < NumPoints - 2; i++)
{
float filteredY = 0f;
for (int j = 0; j < 5; j++)
{
filteredY += coefficients[j] * _points[i - 2 + j].Y;
}
_filteredPoints[i] = new PointF(_points[i].X, filteredY);
}
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
Graphics g = e.Graphics;
// Draw axes
g.DrawLine(Pens.Black, Margin, Margin, Margin, Height - Margin);
g.DrawLine(Pens.Black, Margin, Height - Margin, Width - Margin, Height - Margin);
g.DrawString("0", Font, Brushes.Black, Margin - 10, Height - Margin + 5);
g.DrawString("2π", Font, Brushes.Black, Width - Margin + 5, Height - Margin + 5);
g.DrawString("y", Font, Brushes.Black, Margin - 20, Margin - 15);
g.DrawString("x", Font, Brushes.Black, Width - Margin + 10, Height - Margin + 5);
// Draw sin wave
for (int i = 0; i < NumPoints - 1; i++)
{
g.DrawLine(_sinPen, _points[i], _points[i + 1]);
}
// Draw noise
for (int i = 0; i < NumPoints - 1; i++)
{
g.DrawLine(_noisePen, _points[i].X, _points[i].Y, _points[i + 1].X, _points[i + 1].Y);
}
// Draw filtered wave
for (int i = 0; i < NumPoints - 1; i++)
{
g.DrawLine(_filteredPen, _filteredPoints[i], _filteredPoints[i + 1]);
}
}
}
}
```
该代码生成一个带有噪声的正弦曲线,并使用S-G滤波器对其进行平滑处理。然后,它使用Drawing将原始曲线、噪声曲线和平滑曲线绘制为折线图。您可以在窗体的OnPaint事件中使用此代码来显示绘图。
阅读全文
相关推荐



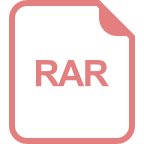
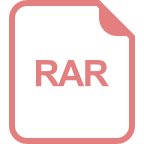
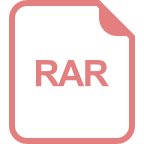
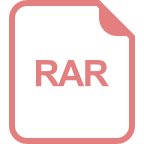
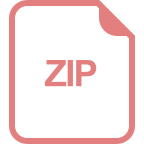
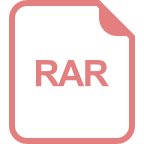
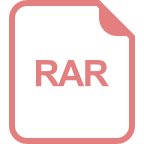
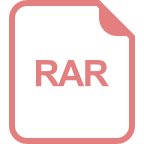
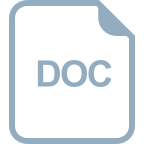
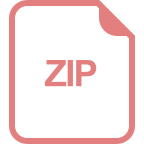
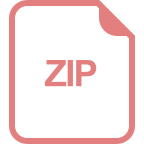
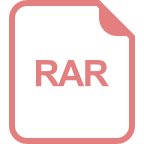
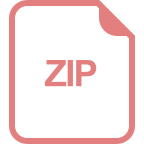