Eth代币转账python实现代码
时间: 2023-07-23 16:47:22 浏览: 328
以下是一个基于Python的Eth代币转账实现代码示例,需要使用web3.py库:
```python
from web3 import Web3
import json
# 1. 连接到以太坊节点
web3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/{your-infura-project-id}'))
# 2. 加载代币合约ABI
with open('abi.json', 'r') as abi_definition:
abi = json.load(abi_definition)
# 3. 获取代币合约
contract_address = '0x1234567890123456789012345678901234567890'
contract = web3.eth.contract(address=contract_address, abi=abi)
# 4. 发送代币转账交易
token_sender_address = '0x1234567890123456789012345678901234567890'
token_sender_private_key = '{your-private-key}'
token_receiver_address = '0x0987654321098765432109876543210987654321'
token_amount = 1000
gas_limit = 100000
gas_price = web3.toWei('20', 'gwei')
# 获取nonce值
nonce = web3.eth.getTransactionCount(token_sender_address)
# 构造交易数据
transaction = {
'nonce': nonce,
'to': contract_address,
'value': 0,
'gas': gas_limit,
'gasPrice': gas_price,
'data': contract.functions.transfer(token_receiver_address, token_amount).buildTransaction({'from': token_sender_address}).hex(),
}
# 对交易进行签名
signed_txn = web3.eth.account.signTransaction(transaction, private_key=token_sender_private_key)
# 发送交易
tx_hash = web3.eth.sendRawTransaction(signed_txn.rawTransaction)
# 等待交易被打包
tx_receipt = web3.eth.waitForTransactionReceipt(tx_hash)
# 打印交易信息
print(f'Transaction sent: {tx_hash.hex()}')
print(f'Transaction receipt: {tx_receipt}')
```
其中,需要将代码中的以下参数替换为实际的值:
- `{your-infura-project-id}`:Infura项目ID
- `abi.json`:代币合约ABI文件路径
- `contract_address`:代币合约地址
- `token_sender_address`:代币发送者地址
- `token_sender_private_key`:代币发送者私钥
- `token_receiver_address`:代币接收者地址
- `token_amount`:代币数量
- `gas_limit`:交易的gas限制
- `gas_price`:交易的gas价格
需要注意的是,这只是一个简单的示例代码,实际使用中需要根据具体情况进行调整和完善。同时,在使用时需要非常小心,以免出现错误导致代币丢失。建议在测试环境中进行充分的测试,确保代码的正确性和安全性。
阅读全文
相关推荐

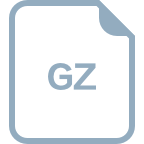











