生成按照顺序读取文件内图片的python代码
时间: 2023-09-15 08:05:40 浏览: 106
### 回答1:
以下是一个读取文件夹中图片的示例代码:
```python
import os
from PIL import Image
# 定义文件夹路径
folder_path = 'path/to/your/folder'
# 获取文件夹中所有图片文件的名称
image_files = [f for f in os.listdir(folder_path) if f.endswith('.jpg') or f.endswith('.png') or f.endswith('.jpeg')]
# 按照文件名排序
image_files.sort()
# 读取图片文件
for image_file in image_files:
image_path = os.path.join(folder_path, image_file)
image = Image.open(image_path)
# 你可以在这里进行相关的图片处理操作
```
这段代码中,我们使用了 `os` 库来读取文件夹中的文件,并使用 `PIL` 库来打开图片文件。代码会读取文件夹中的所有图片文件,并使用 `sort()` 函数按照文件名排序。最后,代码通过一个 for 循环来读取每一张图片。
### 回答2:
按照顺序读取文件内图片的Python代码如下:
```python
import os
def get_ordered_images(folder):
image_files = []
for file in os.listdir(folder):
if file.endswith(".jpg") or file.endswith(".png"):
image_files.append(file)
image_files.sort() # 按字典顺序排序图片文件名
images = []
for file in image_files:
image_path = os.path.join(folder, file)
images.append(image_path)
return images
folder_path = "/path/to/folder" # 文件夹路径
images = get_ordered_images(folder_path) # 获取按顺序的图片路径
for image in images:
# 处理图片,例如显示或保存
print(image)
```
以上代码首先定义了一个函数`get_ordered_images`,用于从给定文件夹中获取按照顺序排列的图片路径。函数首先将文件夹中的所有文件遍历一遍,筛选出以".jpg"或".png"结尾的文件,并存储在`image_files`列表中。然后,对`image_files`进行排序,按字典顺序排列图片文件名,得到按顺序的图片文件列表。最后,遍历按顺序排列的图片文件列表,可以对每一张图片进行相应的处理,例如显示或保存图片。
### 回答3:
下面是一个生成按照顺序读取文件内图片的Python代码:
```python
import os
def get_ordered_images(directory):
# 存储图片的列表
image_list = []
# 遍历文件夹内所有文件
for filename in sorted(os.listdir(directory)):
# 判断文件是否为图片格式
if filename.endswith((".jpg", ".jpeg", ".png")):
# 获取图片的完整路径
image_path = os.path.join(directory, filename)
# 将图片路径添加到列表中
image_list.append(image_path)
return image_list
# 指定文件夹路径
folder_path = '图片文件夹路径'
# 获取按顺序读取的图片列表
images = get_ordered_images(folder_path)
# 遍历图片列表并读取图片
for image_path in images:
# 在这里编写读取图片的操作,例如使用PIL库进行读取和处理
# 例如:image = PIL.Image.open(image_path)
# 在这里可以对图片进行任意操作,例如显示、保存等
```
上述代码中,我们首先定义了一个函数`get_ordered_images`,该函数接受一个文件夹路径作为参数,并返回按顺序读取的图片列表。函数使用`os.listdir`遍历文件夹内的所有文件,并判断文件是否为图片格式。如果是图片格式,则将图片的完整路径添加到图片列表中。最后,我们遍历图片列表并读取每张图片。
请注意,需要将代码中的`'图片文件夹路径'`修改为实际的图片文件夹路径。另外,根据实际需求,你可能需要使用适当的库来读取和处理图片,例如使用PIL库(`pillow`)进行读取和处理。
阅读全文
相关推荐
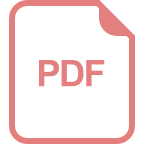
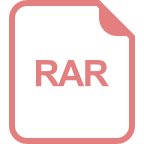
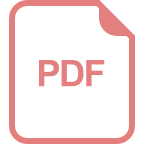
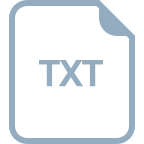
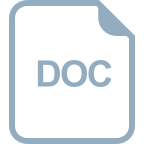
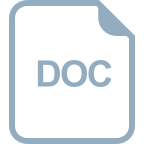












