帮我写个使用winhttpApi访问https://www.baidu.com且导入证书的c++例子 并计算请求时间
时间: 2023-08-16 09:07:00 浏览: 160
好的,下面是一个使用winhttpApi访问https://www.baidu.com并导入证书的c++例子,并计算请求时间的代码:
```c++
#include <iostream>
#include <windows.h>
#include <winhttp.h>
#include <time.h>
#pragma comment(lib, "winhttp.lib")
int main()
{
HINTERNET hSession = NULL, hConnect = NULL, hRequest = NULL;
DWORD dwSize = 0;
DWORD dwDownloaded = 0;
LPSTR pszOutBuffer;
BOOL bResults = FALSE;
DWORD dwStatusCode = 0;
clock_t start_time, end_time;
// Initialize WinHTTP session
hSession = WinHttpOpen(L"WinHTTP Example/1.0", WINHTTP_ACCESS_TYPE_DEFAULT_PROXY, WINHTTP_NO_PROXY_NAME, WINHTTP_NO_PROXY_BYPASS, 0);
if (hSession == NULL)
{
std::cout << "Error " << GetLastError() << " in WinHttpOpen" << std::endl;
goto done;
}
// Specify an HTTPS server
hConnect = WinHttpConnect(hSession, L"www.baidu.com", INTERNET_DEFAULT_HTTPS_PORT, 0);
if (hConnect == NULL)
{
std::cout << "Error " << GetLastError() << " in WinHttpConnect" << std::endl;
goto done;
}
// Open request
hRequest = WinHttpOpenRequest(hConnect, L"GET", NULL, NULL, WINHTTP_NO_REFERER, WINHTTP_DEFAULT_ACCEPT_TYPES, WINHTTP_FLAG_SECURE);
if (hRequest == NULL)
{
std::cout << "Error " << GetLastError() << " in WinHttpOpenRequest" << std::endl;
goto done;
}
// Import certificate
HCERTSTORE hCertStore = CertOpenSystemStore(NULL, L"MY");
if (hCertStore == NULL)
{
std::cout << "Error " << GetLastError() << " in CertOpenSystemStore" << std::endl;
goto done;
}
PCCERT_CONTEXT pCertContext = CertFindCertificateInStore(hCertStore, X509_ASN_ENCODING | PKCS_7_ASN_ENCODING, 0, CERT_FIND_SUBJECT_STR, L"www.baidu.com", NULL);
if (pCertContext == NULL)
{
std::cout << "Error " << GetLastError() << " in CertFindCertificateInStore" << std::endl;
goto done;
}
bResults = WinHttpSetOption(hRequest, WINHTTP_OPTION_CLIENT_CERT_CONTEXT, (LPVOID)pCertContext, sizeof(CERT_CONTEXT));
if (!bResults)
{
std::cout << "Error " << GetLastError() << " in WinHttpSetOption" << std::endl;
goto done;
}
// Send request
start_time = clock();
bResults = WinHttpSendRequest(hRequest, WINHTTP_NO_ADDITIONAL_HEADERS, 0, WINHTTP_NO_REQUEST_DATA, 0, 0, 0);
if (!bResults)
{
std::cout << "Error " << GetLastError() << " in WinHttpSendRequest" << std::endl;
goto done;
}
// Receive response
bResults = WinHttpReceiveResponse(hRequest, NULL);
if (!bResults)
{
std::cout << "Error " << GetLastError() << " in WinHttpReceiveResponse" << std::endl;
goto done;
}
// Check status code
dwSize = sizeof(dwStatusCode);
bResults = WinHttpQueryHeaders(hRequest, WINHTTP_QUERY_STATUS_CODE | WINHTTP_QUERY_FLAG_NUMBER, NULL, &dwStatusCode, &dwSize, NULL);
if (!bResults)
{
std::cout << "Error " << GetLastError() << " in WinHttpQueryHeaders" << std::endl;
goto done;
}
if (dwStatusCode != HTTP_STATUS_OK)
{
std::cout << "Error: status code " << dwStatusCode << std::endl;
goto done;
}
// Read response
do
{
// Check for available data
dwSize = 0;
bResults = WinHttpQueryDataAvailable(hRequest, &dwSize);
if (!bResults)
{
std::cout << "Error " << GetLastError() << " in WinHttpQueryDataAvailable" << std::endl;
goto done;
}
// Allocate space for buffer
pszOutBuffer = new char[dwSize + 1];
if (!pszOutBuffer)
{
std::cout << "Error out of memory" << std::endl;
goto done;
}
// Read data
ZeroMemory(pszOutBuffer, dwSize + 1);
bResults = WinHttpReadData(hRequest, (LPVOID)pszOutBuffer, dwSize, &dwDownloaded);
if (!bResults)
{
std::cout << "Error " << GetLastError() << " in WinHttpReadData" << std::endl;
goto done;
}
// Print data
std::cout << pszOutBuffer;
// Free the memory allocated to the buffer.
delete[] pszOutBuffer;
} while (dwSize > 0);
end_time = clock();
double elapsed_time = (double)(end_time - start_time) / CLOCKS_PER_SEC;
std::cout << "Elapsed time: " << elapsed_time << " seconds" << std::endl;
done:
// Clean up
if (hRequest) WinHttpCloseHandle(hRequest);
if (hConnect) WinHttpCloseHandle(hConnect);
if (hSession) WinHttpCloseHandle(hSession);
if (pCertContext) CertFreeCertificateContext(pCertContext);
if (hCertStore) CertCloseStore(hCertStore, 0);
return 0;
}
```
该代码首先初始化一个WinHTTP会话,然后指定要访问的HTTPS服务器。然后打开请求并导入证书,发送请求并接收响应,最后计算请求时间。请注意,代码中的证书名称应更改为您的证书。
阅读全文
相关推荐


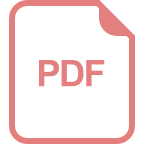
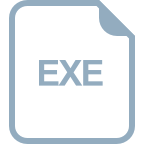
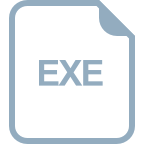
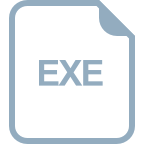
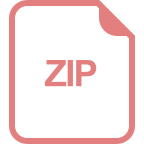
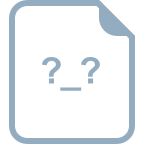
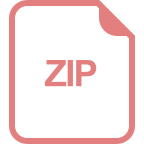
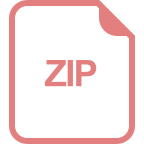
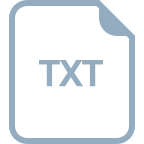
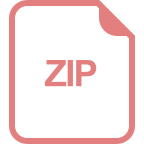
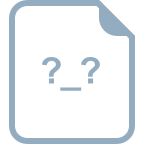
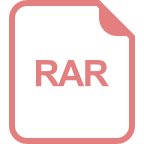
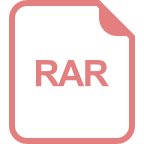
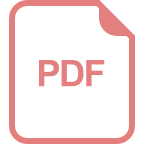