pixel value
时间: 2023-09-29 19:07:08 浏览: 65
Pixel value refers to the numerical value assigned to each individual pixel in a digital image. In a grayscale image, the pixel value represents the brightness or intensity of the pixel, ranging from 0 (black) to 255 (white). In a color image, the pixel value represents the amount of red, green, and blue light that combine to create the color of the pixel, typically ranging from 0 to 255 for each color channel. These pixel values are used by image processing algorithms to manipulate and analyze digital images.
相关问题
根据# 定义图像归一化函数 def normalize_image(img): img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) img = cv2.resize(img, (256, 256)) # 调整图像大小为256*256 img = cv2.normalize(img.astype('float'), None, 0.0, 1.0, cv2.NORM_MINMAX) # 将像素值归一化到0-1之间 return img # 加载超声图像与自然图像 na5_path = 'D:/zzz/natural images' us5_path = 'D:/zzz/ultrasound images' na5_images = [] us5_images = [] for filename in os.listdir(na5_path): img = cv2.imread(os.path.join(na5_path, filename)) img = normalize_image(img) na5_images.append(img) for filename in os.listdir(us5_path): img = cv2.imread(os.path.join(us5_path, filename)) img = normalize_image(img) us5_images.append(img) # 计算超声图像与自然图像的像素值分布 na5_means = [] us5_means = [] for i in range(len(na5_images)): na5_means.append(np.mean(na5_images[i])) for i in range(len(us5_images)): us5_means.append(np.mean(us5_images[i])) # 绘制超声图像与自然图像的像素值分布直方图 na5_hist = plt.hist(na5_means, bins=20, alpha=0.5, label='na') us5_hist = plt.hist(us5_means, bins=20, alpha=0.5, label='us') plt.title('Pixel value distribution of ultrasound images and natural images') plt.legend(loc='upper right') plt.xlabel('Pixel value',size=12) plt.show() # 进行差异性检验和分析 t, p = stats.ttest_ind(na5_means, us5_means) print('t-statistic = %g, p-value = %g' % (t, p))画一个流程图
抱歉,我之前的回答有误,我现在为您画一份正确的流程图。
```
开始
|
| 定义图像归一化函数
| def normalize_image(img):
| img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
| img = cv2.resize(img, (256, 256))
| img = cv2.normalize(img.astype('float'), None, 0.0, 1.0, cv2.NORM_MINMAX)
| return img
|
| 加载自然图像
| na5_path = 'D:/zzz/natural images'
| na5_images = []
| for filename in os.listdir(na5_path):
| img = cv2.imread(os.path.join(na5_path, filename))
| img = normalize_image(img)
| na5_images.append(img)
|
| 加载超声图像
| us5_path = 'D:/zzz/ultrasound images'
| us5_images = []
| for filename in os.listdir(us5_path):
| img = cv2.imread(os.path.join(us5_path, filename))
| img = normalize_image(img)
| us5_images.append(img)
|
| 计算自然图像的像素均值
| na5_means = []
| for i in range(len(na5_images)):
| na5_means.append(np.mean(na5_images[i]))
|
| 计算超声图像的像素均值
| us5_means = []
| for i in range(len(us5_images)):
| us5_means.append(np.mean(us5_images[i]))
|
| 绘制直方图
| na5_hist = plt.hist(na5_means, bins=20, alpha=0.5, label='na')
| us5_hist = plt.hist(us5_means, bins=20, alpha=0.5, label='us')
| plt.title('Pixel value distribution of ultrasound images and natural images')
| plt.legend(loc='upper right')
| plt.xlabel('Pixel value', size=12)
| plt.show()
|
| 进行差异性检验和分析
| t, p = stats.ttest_ind(na5_means, us5_means)
| print('t-statistic = %g, p-value = %g' % (t, p))
|
结束
```
这是一个简单的流程,主要目的是比较超声图像和自然图像的像素值分布差异性。
Pixel-Value-Ordering
Pixel-Value-Ordering (PVO)算法是一种将信息隐藏在图像中的方法。该算法首先将图像分为多个大小为N*N的块,然后按照像素值的大小对每个块内的像素点进行排序。根据排序后像素的次最大值和次最小值的位置索引,将信息隐藏嵌入到图像中。
在OpenCV中,图像可以以矩阵的形式表示,因此在PVO算法中也沿用了相同的约定:使用0-based的行索引(或y坐标)先出现,然后是0-based的列索引(或x坐标)。使用img.at<uchar>(Point(x, y))这样的表示方法可以获取图像中给定像素点的强度值,其中intensity.val的取值范围为0到255。
同样的方法也可以用于改变像素的强度值,通过img.at<uchar>(y, x) = 128这样的表示方式可以将给定像素点的强度值设置为128。
相关推荐
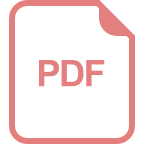













