利用适当的容器类实现电话簿类 PhoneBook,要求如下。 (1) 联系人信息包括:姓名,电话号码。 (2)支持添加新联系人、删除联系人。 (3)支持双向查找:给定姓名查找电话号码,给定电话号码查找姓名。 (4)支持电话簿导出到文本文件,导出的文件每行存储一条联系人记录,姓名和电话号码之间用空白分隔。 (5) 支持从文本文件导入电话簿;文件中每行有一条联系人记录,姓名和电话号码之间用空白分隔。
时间: 2024-02-25 14:59:00 浏览: 22
好的,我可以帮你解答这个问题。你可以使用 C++ 的 STL 中的 map 类来实现 PhoneBook 类。下面是 PhoneBook 类的基本实现:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <map>
using namespace std;
class PhoneBook {
public:
void addContact(const string& name, const string& number) {
contacts[name] = number;
reverseContacts[number] = name;
}
void removeContact(const string& name) {
if (contacts.count(name) > 0) {
string number = contacts[name];
contacts.erase(name);
reverseContacts.erase(number);
}
}
string findNumber(const string& name) const {
if (contacts.count(name) > 0)
return contacts.at(name);
else
return "Not Found";
}
string findName(const string& number) const {
if (reverseContacts.count(number) > 0)
return reverseContacts.at(number);
else
return "Not Found";
}
void exportToFile(const string& filename) const {
ofstream outfile(filename);
if (outfile.is_open()) {
for (const auto& contact : contacts) {
outfile << contact.first << " " << contact.second << endl;
}
outfile.close();
} else {
cout << "Unable to open file for export" << endl;
}
}
void importFromFile(const string& filename) {
ifstream infile(filename);
if (infile.is_open()) {
string name, number;
while (infile >> name >> number) {
addContact(name, number);
}
infile.close();
} else {
cout << "Unable to open file for import" << endl;
}
}
private:
map<string, string> contacts;
map<string, string> reverseContacts;
};
```
这个实现中,我们使用了两个 map 容器来存储联系人信息。其中,contacts 容器用来根据姓名查找电话号码,reverseContacts 容器用来根据电话号码查找姓名。
在 PhoneBook 类中,我们实现了以下几个方法:
- `addContact`:用于添加联系人信息。
- `removeContact`:用于删除联系人信息。
- `findNumber`:用于根据姓名查找电话号码。
- `findName`:用于根据电话号码查找姓名。
- `exportToFile`:用于将电话簿导出到文本文件。
- `importFromFile`:用于从文本文件导入电话簿。
你可以根据自己的需求来使用这些方法。希望对你有所帮助!
相关推荐
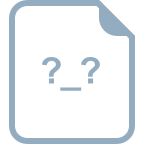















