利用opencv查看文件夹下文件夹中的图片实现人脸识别并显示出它的名字
时间: 2023-08-15 15:07:17 浏览: 50
好的,我来给你讲一下具体的实现步骤。
1. 导入必要的库
首先需要导入opencv库和os库。
```python
import cv2
import os
```
2. 加载人脸识别模型
使用opencv提供的人脸识别模型进行人脸识别任务。
```python
face_cascade = cv2.CascadeClassifier('haarcascade_frontalface_default.xml')
```
3. 加载图片并进行人脸检测
遍历文件夹下的每一张图片,使用opencv提供的人脸检测方法检测人脸,并将检测到的人脸保存在一个列表中。
```python
def detect_face(img_path):
img = cv2.imread(img_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5)
return faces
```
4. 显示人脸和名字
根据检测到的人脸位置,将对应的名字显示在图片上。
```python
def show_face_name(img_path, name):
img = cv2.imread(img_path)
faces = detect_face(img_path)
for (x, y, w, h) in faces:
cv2.rectangle(img, (x, y), (x+w, y+h), (0, 255, 0), 2)
cv2.putText(img, name, (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.9, (36,255,12), 2)
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
5. 遍历文件夹并进行人脸识别
使用os库遍历文件夹下的所有文件夹和图片,并调用上述方法进行人脸识别和显示。
```python
root_path = 'your_folder_path'
for dir_name in os.listdir(root_path):
dir_path = os.path.join(root_path, dir_name)
if os.path.isdir(dir_path):
for img_name in os.listdir(dir_path):
img_path = os.path.join(dir_path, img_name)
faces = detect_face(img_path)
if len(faces) > 0:
show_face_name(img_path, dir_name)
```
这样就完成了人脸识别并显示名字的任务。
相关推荐
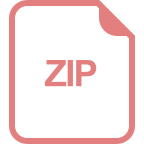
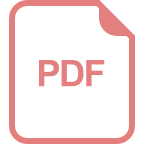
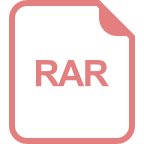














