C语言载入txt文件
时间: 2023-07-04 10:12:17 浏览: 60
您可以使用C语言中的标准库函数fopen()和fscanf()来载入txt文件。
下面是一个简单的示例代码:
```c
#include <stdio.h>
int main() {
FILE *fp;
char filename[] = "example.txt";
int num1, num2;
fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s\n", filename);
return 1;
}
fscanf(fp, "%d %d", &num1, &num2);
printf("The first number is %d and the second number is %d\n", num1, num2);
fclose(fp);
return 0;
}
```
在这个示例中,我们打开了名为example.txt的文件,使用fscanf()函数读取了文件中的前两个整数,并将它们打印到控制台上。最后,我们使用fclose()函数关闭了文件。
请注意,如果文件打开失败,我们会收到一个错误消息并退出程序。
相关问题
c语言游戏状态的文件保存载入
在C语言中,可以使用文件I/O操作来保存和载入游戏状态。
首先,需要定义一个结构体来存储游戏状态的各个变量,例如:
```c
struct game_state {
int level;
int score;
char player_name[20];
// 其他变量
};
```
然后,可以使用fopen()函数打开一个文件,使用fprintf()函数将游戏状态写入文件,使用fscanf()函数从文件中读取游戏状态。例如:
```c
void save_game_state(struct game_state state) {
FILE *fp;
fp = fopen("game_state.txt", "w");
fprintf(fp, "%d %d %s", state.level, state.score, state.player_name);
fclose(fp);
}
struct game_state load_game_state() {
struct game_state state;
FILE *fp;
fp = fopen("game_state.txt", "r");
fscanf(fp, "%d %d %s", &state.level, &state.score, state.player_name);
fclose(fp);
return state;
}
```
注意,需要在编译时加上 -lm 参数,以链接数学库。完整的代码示例如下:
```c
#include <stdio.h>
struct game_state {
int level;
int score;
char player_name[20];
};
void save_game_state(struct game_state state) {
FILE *fp;
fp = fopen("game_state.txt", "w");
fprintf(fp, "%d %d %s", state.level, state.score, state.player_name);
fclose(fp);
}
struct game_state load_game_state() {
struct game_state state;
FILE *fp;
fp = fopen("game_state.txt", "r");
fscanf(fp, "%d %d %s", &state.level, &state.score, state.player_name);
fclose(fp);
return state;
}
int main() {
struct game_state state = {5, 100, "Alice"};
save_game_state(state);
struct game_state loaded_state = load_game_state();
printf("Loaded game state: level=%d, score=%d, player_name=%s\n",
loaded_state.level, loaded_state.score, loaded_state.player_name);
return 0;
}
```
使用c++读取大小为40G的txt文件,并将其中的数据进行升序排序,并输出到指定txt文件之中
### 回答1:
读取和排序40G的数据需要考虑内存使用和效率问题,可以采用外部排序(External Sorting)的方法。具体步骤如下:
1. 将40G的txt文件分成若干个小文件,每个小文件可以完全载入内存进行排序。
2. 对每个小文件进行排序,常见的排序算法有快速排序(QuickSort)、归并排序(MergeSort)等。这里推荐使用归并排序,因为归并排序在外部排序中表现更好。
3. 对每个小文件排序后,将它们合并成一个大文件。可以使用归并排序的思想,将每个小文件的第一个元素进行比较,取最小值放入输出文件中,然后再从该元素所在的小文件中取下一个元素进行比较,直到所有小文件的元素都被处理完。
4. 重复步骤3,直到所有小文件都被处理完,最终得到的输出文件即为升序排序后的结果。
代码示例:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
#include <queue>
using namespace std;
const int MAX_BLOCK_SIZE = 1024 * 1024 * 1024; // 每个小文件的最大大小,这里设置为1GB
const int MAX_LINE_SIZE = 1024; // 每行数据的最大长度
struct Block {
char* data;
int size;
int offset; // 在原文件中的偏移量
Block(char* data, int size, int offset) : data(data), size(size), offset(offset) {}
~Block() {
delete[] data;
}
};
struct CompareBlock {
bool operator()(const Block& a, const Block& b) const {
// 比较每个块的第一行数据,用于归并排序
return atoi(a.data) > atoi(b.data);
}
};
void sortAndWriteToFile(Block& block, const string& filename) {
vector<string> lines;
char* p = strtok(block.data, "\n");
while (p != nullptr) {
lines.push_back(p);
p = strtok(nullptr, "\n");
}
sort(lines.begin(), lines.end());
ofstream fout(filename, ios::app);
for (const auto& line : lines) {
fout << line << endl;
}
}
void mergeAndWriteToFile(vector<string>& lines, const string& filename) {
sort(lines.begin(), lines.end());
ofstream fout(filename, ios::app);
for (const auto& line : lines) {
fout << line << endl;
}
}
void externalSort(const string& inputFilename, const string& outputFilename) {
ifstream fin(inputFilename);
if (!fin.is_open()) {
cerr << "Failed to open file: " << inputFilename << endl;
return;
}
// 第一遍扫描,将文件分成若干个小文件
vector<string> filenames;
char* blockData = new char[MAX_BLOCK_SIZE];
int blockOffset = 0;
int blockSize = 0;
while (fin.getline(blockData + blockSize, MAX_LINE_SIZE)) {
int lineSize = strlen(blockData + blockSize);
blockSize += lineSize + 1;
if (blockSize > MAX_BLOCK_SIZE) {
// 当前块已满,将其写入文件
string filename = "block_" + to_string(filenames.size()) + ".txt";
ofstream fout(filename);
fout.write(blockData, blockSize);
fout.close();
filenames.push_back(filename);
blockOffset += blockSize;
blockSize = 0;
}
}
if (blockSize > 0) {
// 将最后一块写入文件
string filename = "block_" + to_string(filenames.size()) + ".txt";
ofstream fout(filename);
fout.write(blockData, blockSize);
fout.close();
filenames.push_back(filename);
}
delete[] blockData;
// 第二遍扫描,对每个小文件进行排序,并将排序结果写入输出文件
priority_queue<Block, vector<Block>, CompareBlock> pq;
for (const auto& filename : filenames) {
ifstream fin(filename);
if (!fin.is_open()) {
cerr << "Failed to open file: " << filename << endl;
continue;
}
fin.seekg(0, ios::end);
int fileSize = fin.tellg();
fin.seekg(0, ios::beg);
char* data = new char[fileSize];
fin.read(data, fileSize);
fin.close();
pq.emplace(data, fileSize, blockOffset);
blockOffset += fileSize;
}
while (!pq.empty()) {
Block block = pq.top();
pq.pop();
sortAndWriteToFile(block, outputFilename);
if (block.size > MAX_BLOCK_SIZE) {
// 如果当前块太大,则拆分成若干个小块,并重新加入优先队列
int numBlocks = block.size / MAX_BLOCK_SIZE;
int lastBlockSize = block.size % MAX_BLOCK_SIZE;
int blockStart = 0;
for (int i = 0; i < numBlocks; i++) {
char* data = new char[MAX_BLOCK_SIZE];
memcpy(data, block.data + blockStart, MAX_BLOCK_SIZE);
pq.emplace(data, MAX_BLOCK_SIZE, block.offset + blockStart);
blockStart += MAX_BLOCK_SIZE;
}
if (lastBlockSize > 0) {
char* data = new char[lastBlockSize];
memcpy(data, block.data + blockStart, lastBlockSize);
pq.emplace(data, lastBlockSize, block.offset + blockStart);
}
} else {
delete block.data;
}
}
// 第三遍扫描,将每个小文件中的数据合并成一个大文件
vector<string> blockLines(filenames.size());
vector<ifstream> finList(filenames.size());
for (int i = 0; i < filenames.size(); i++) {
finList[i].open(filenames[i]);
if (!finList[i].is_open()) {
cerr << "Failed to open file: " << filenames[i] << endl;
continue;
}
getline(finList[i], blockLines[i]);
}
while (true) {
// 取每个块中的最小值
int minIndex = -1;
for (int i = 0; i < blockLines.size(); i++) {
if (finList[i].eof()) {
continue;
}
if (minIndex == -1 || blockLines[i] < blockLines[minIndex]) {
minIndex = i;
}
}
if (minIndex == -1) {
break;
}
mergeAndWriteToFile(blockLines, outputFilename);
getline(finList[minIndex], blockLines[minIndex]);
}
}
int main() {
externalSort("input.txt", "output.txt");
return 0;
}
```
上述代码中,我们首先将大文件分成若干个小文件,每个小文件的大小不超过1GB,然后对每个小文件进行排序,并将排序结果写入输出文件。接着,我们将每个小文件的第一行数据加入一个优先队列中,并按照归并排序的思想将它们逐个取出,取出的同时从相应的小文件中取下一个元素进行比较,直到所有小文件的元素都被处理完。最终得到的输出文件即为升序排序后的结果。
### 回答2:
要使用C语言读取大小为40G的txt文件并进行排序,需要采取一些策略来处理文件的大小和排序的效率。以下是一种可能的实现:
1. 首先,确定是否有足够的内存来处理40G的文件。如果内存不足,可以考虑使用外部排序算法,将文件划分为更小的部分进行排序并最后合并。
2. 创建一个用于存储文件数据的缓冲区。由于文件较大,可以选择多次读取文件的一部分数据到缓冲区中进行排序。
3. 使用文件指针打开输入txt文件和输出txt文件。可以使用fopen()函数打开文件,并用fscanf()函数逐行读取数据。
4. 将读取到的数据存储在数组中。
5. 对数组进行排序。可以使用标准库中的qsort()函数进行快速排序,或自己实现其他排序算法。
6. 将排序后的数据写入输出txt文件。使用fprintf()函数将数组中的排序后的数据逐行写入到输出文件中。
7. 重复步骤4-6,直到读取完整个输入文件。
8. 关闭输入和输出文件, 使用fclose()函数关闭文件。
需要注意的是,在处理大文件时,可能需要使用一些优化技巧,例如使用多线程或使用磁盘缓存来提高读写效率。同时,在进行文件处理时,也要注意错误处理和内存泄漏的情况。
以上就是一个简单的实现思路,根据实际情况和需求可能需要进行更详细和复杂的处理。
### 回答3:
要使用C语言读取大小为40G的txt文件并进行排序是一项庞大的任务。由于文本文件大小超过了内存的限制,我们不能一次性将整个文件读入内存并进行排序。
为了处理这个问题,我们可以采用外部排序算法,其中涉及将大文件划分为小块,并分别对这些小块进行排序。然后,我们可以使用归并排序算法将所有小块合并到一个有序文件中。
首先,我们需要将大文件划分为多个小文件,而不是一次性读取整个文件。我们可以使用缓冲区,每次只读取一部分数据,然后将其写入新的临时文件。在读取和写入期间,我们可以使用快速排序或堆排序等算法对数据进行排序。
在排序完成后,我们可以使用归并排序算法合并所有的临时文件。归并排序算法更适合处理大量数据,它将多个有序文件合并为一个大文件。
最后,我们将排序后的数据写入指定的txt文件中。
这个任务需要计算机性能较强的设备、大量的时间和存储空间。我建议在处理大文件时使用适当的硬件和利用并行计算的优势,以加快处理速度。
总的来说,使用C语言读取并排序大型txt文件是一项挑战性的任务,需要使用外部排序和归并排序算法,以及较强的硬件和适当的计算资源。
相关推荐
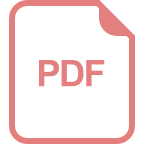
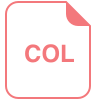
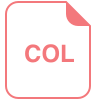
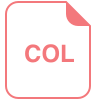
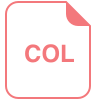
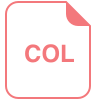




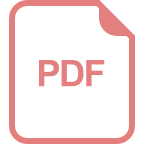
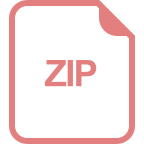
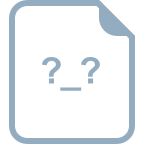
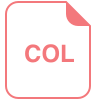