springboot+vue实现文件下载功能,写出代码
时间: 2023-11-09 08:05:34 浏览: 49
好的,以下是一个简单的示例代码:
后端 Spring Boot:
```java
@RestController
public class FileDownloadController {
@GetMapping("/download")
public ResponseEntity<Resource> downloadFile() {
// 从服务器本地获取文件
Resource resource = new FileSystemResource("path/to/file");
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.contentLength(resource.contentLength())
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
}
}
```
前端 Vue:
```html
<template>
<div>
<button @click="downloadFile">下载文件</button>
</div>
</template>
<script>
import axios from 'axios';
export default {
methods: {
downloadFile() {
axios({
url: '/download',
method: 'GET',
responseType: 'blob'
}).then(response => {
const url = window.URL.createObjectURL(new Blob([response.data]));
const link = document.createElement('a');
link.href = url;
link.setAttribute('download', 'file.txt');
document.body.appendChild(link);
link.click();
});
}
}
}
</script>
```
在这个示例中,我们在后端使用了 Spring Boot 的 `Resource` 类来获取本地文件资源,然后在响应头中设置了下载文件的名称和类型。在前端,我们使用了 Axios 库来发送 GET 请求,并将响应的数据转换成 Blob 对象,最后创建一个下载链接并点击下载。
相关推荐
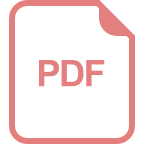
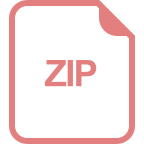
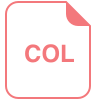









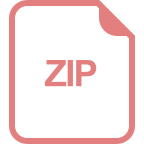
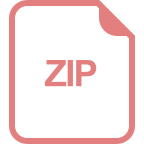
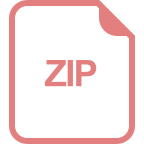
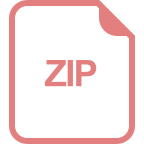
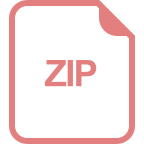