编写c++程序,用二进制方式打开指定的一个文件,在每一行前加行号。
时间: 2023-12-06 22:44:15 浏览: 173
以下是实现该功能的 C++ 代码:
```cpp
#include <iostream>
#include <fstream>
using namespace std;
int main() {
ifstream infile("filename.txt", ios::binary); // 以二进制方式打开文件
ofstream outfile("output.txt"); // 创建输出文件
int line_num = 1; // 记录行号
// 逐行读取文件内容
string line;
while (getline(infile, line)) {
// 在每行前加上行号并写入输出文件
outfile << line_num << ": " << line << endl;
line_num++;
}
infile.close(); // 关闭输入文件
outfile.close(); // 关闭输出文件
return 0;
}
```
其中,需要将 `filename.txt` 替换为指定的文件名,程序会将处理后的结果输出到 `output.txt` 文件中。
相关问题
C++编写程序,用二进制方式打开指定的一个文件,在每一行前加行号
下面是一个简单的C++程序,可以实现在文件的每一行前加上行号的操作:
```cpp
#include <iostream>
#include <fstream>
#include <iomanip>
using namespace std;
int main()
{
string filename = "example.txt"; // 指定文件名
ifstream fin(filename, ios::binary); // 以二进制方式打开文件
ofstream fout("output.txt", ios::binary); // 打开输出文件
if (!fin) {
cerr << "Failed to open input file!" << endl;
return -1;
}
if (!fout) {
cerr << "Failed to open output file!" << endl;
return -1;
}
int lineno = 1;
string line;
while (getline(fin, line)) { // 读取文件每一行
fout << setw(3) << setfill('0') << lineno++ << " "; // 输出行号,宽度为3,不足部分用0填充
fout.write(line.c_str(), line.size()); // 输出行内容
fout << endl; // 换行
}
fin.close();
fout.close();
return 0;
}
```
这个程序使用了 `ifstream` 和 `ofstream` 来打开文件,其中 `ifstream` 是以二进制方式打开指定的文件,而 `ofstream` 则是打开输出文件。程序先检查了文件是否成功打开,然后使用 `getline` 函数逐行读取文件内容,同时输出行号和行内容到输出文件中。最后关闭文件并返回0表示程序正常结束。
用c++编写程序,用二进制方式打开指定的一个文件,在每一行前加行号
好的,我可以帮你完成这个任务。以下是一个可能的解决方案:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
string filename = "example.txt"; // 指定文件名
ifstream file(filename, ios::binary); // 以二进制方式打开文件
if (!file.is_open()) // 判断文件是否打开成功
{
cerr << "Error: could not open file " << filename << endl;
return 1;
}
int line_num = 1; // 初始化行号为 1
string line;
while (getline(file, line)) // 逐行读取文件内容
{
cout << line_num << ": " << line << endl; // 在每一行前加行号并输出
line_num++; // 行号加 1
}
file.close(); // 关闭文件
return 0;
}
```
请注意,上述代码仅适用于 ASCII 编码的文本文件。如果文件内容包含其他类型的数据(例如图片、视频等),则需要使用不同的方法处理。此外,如果文件中包含非 ASCII 字符,可能需要进行编码转换以确保正确处理。
阅读全文
相关推荐
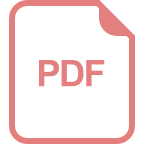
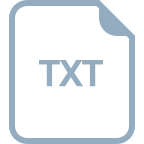










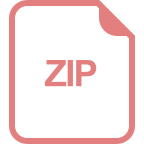
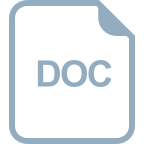
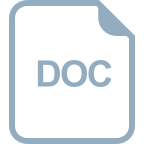
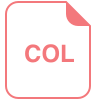