用结构体表示日期(年、月、日),从键盘输入一个日期,计算该日期在当年中是第几天,注意闰年。
时间: 2023-12-12 13:32:56 浏览: 81
好的,我可以帮你解答这个问题。
首先,我们可以使用一个结构体来表示日期,其中包含年、月和日的成员变量。以下是一个示例的结构体定义:
```c
struct Date {
int year;
int month;
int day;
};
```
然后,我们可以从键盘输入一个日期,并将其存储到一个日期结构体变量中。你可以使用`scanf`函数来实现输入操作。例如:
```c
struct Date date;
printf("请输入日期(年 月 日):");
scanf("%d %d %d", &date.year, &date.month, &date.day);
```
接下来,我们需要编写一个函数来计算给定日期在当年中是第几天。我们可以使用一个数组来存储每个月份对应的天数,考虑到闰年的情况。以下是一个示例的计算函数:
```c
int dayOfYear(struct Date date) {
int daysInMonth[] = {31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31};
int dayCount = date.day;
// 考虑闰年的情况
if ((date.year % 4 == 0 && date.year % 100 != 0) || date.year % 400 == 0) {
daysInMonth[1] = 29;
}
for (int i = 0; i < date.month - 1; i++) {
dayCount += daysInMonth[i];
}
return dayCount;
}
```
最后,我们可以在主函数中调用上述函数,并打印出计算结果:
```c
int main() {
struct Date date;
printf("请输入日期(年 月 日):");
scanf("%d %d %d", &date.year, &date.month, &date.day);
int dayCount = dayOfYear(date);
printf("该日期在当年中是第 %d 天\n", dayCount);
return 0;
}
```
这样,当你从键盘输入一个日期后,程序会计算出该日期在当年中是第几天,并将结果打印出来。希望能对你有所帮助!如果你还有其他问题,请继续提问。
相关推荐
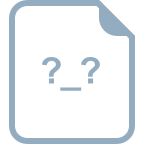
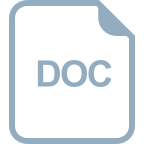
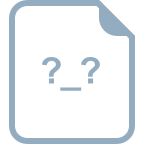
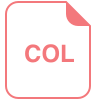
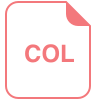
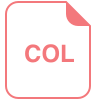
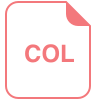
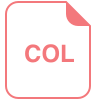









