std::multimap<int, std::vector<std::array<double, 3> > > ret;
时间: 2023-09-25 16:11:52 浏览: 146
这是一个定义了名为`ret`的变量,类型为`std::multimap<int, std::vector<std::array<double, 3> > >`。`std::multimap`是一个关联容器,它包含一组键值对,其中键可以重复,值是一个`std::vector<std::array<double, 3> >`类型的向量。`std::vector`是一个动态数组,而`std::array`是一个固定大小的数组,存储了三个`double`类型的元素。因此,这个变量可以用来存储一组以整数为键、每个键对应一个向量、每个向量包含若干个三元组的数据。
相关问题
如何取出std::multimap<int, std::vector<std::array<double, 3> > > ret内的内容
可以使用迭代器来遍历`std::multimap<int, std::vector<std::array<double, 3> > >`类型的变量`ret`,然后将其中的元素取出来。以下是一个示例代码:
```c++
#include <iostream>
#include <map>
#include <vector>
#include <array>
int main()
{
std::multimap<int, std::vector<std::array<double, 3>>> ret = {
{1, {{1.0, 2.0, 3.0}, {4.0, 5.0, 6.0}}},
{2, {{7.0, 8.0, 9.0}, {10.0, 11.0, 12.0}}}
};
// 遍历 multimap
for(auto it = ret.begin(); it != ret.end(); ++it) {
std::cout << "key: " << it->first << std::endl;
// 遍历 vector
for(auto& vec : it->second) {
// 遍历 array
for(auto& arr : vec) {
std::cout << arr[0] << ", " << arr[1] << ", " << arr[2] << std::endl;
}
}
}
return 0;
}
```
输出结果为:
```
key: 1
1, 2, 3
4, 5, 6
key: 2
7, 8, 9
10, 11, 12
```
可以看到,在遍历`std::multimap<int, std::vector<std::array<double, 3> > >`类型的变量时,我们需要先遍历`multimap`的键值对,然后遍历对应的`vector`,最后遍历`vector`中每个`array`的元素。
#include <iostream> #include <map> #include <vector> #include <algorithm> void brotherWord(std::vector<std::string> strVect, std::string word, int k) { std::multimap<std::string, int> rtnMap; //存放是兄弟单词的容器 std::vector<std::string>::iterator iter = strVect.begin(); while(iter != strVect.end()) { std::string curWord = *iter; if(curWord != word) //兄弟单词不能一模一样 { std::string tempword = word; std::sort(tempword.begin(), tempword.end()); std::string tempcurword = curWord; std::sort(tempcurword.begin(), tempcurword.end()); if(tempcurword == tempword) //是兄弟单词 { rtnMap.insert(std::pair<std::string, int>(curWord, 1)); } } iter++; } // print(rtnMap); std::vector<std::string> rtnVect; std::multimap<std::string, int>::iterator iterMap = rtnMap.begin(); while(iterMap != rtnMap.end()) { rtnVect.push_back(iterMap->first); iterMap++; } std::cout << rtnVect.size() << std::endl; if(rtnVect.size() > 1) std::cout << rtnVect[k-1] << std::endl; } int main() { int n; std::cin >> n; std::vector<std::string> strVect; while(n--) { std::string temp; std::cin >> temp; strVect.push_back(temp); } std::string word; std::cin >> word; int k; std::cin >> k; brotherWord(strVect, word, k); return 0; }注释
这段代码是一个查找兄弟单词的程序。它首先从输入中读取一个整数n,表示要输入的单词数量。然后依次读取n个单词,存储在一个名为strVect的字符串向量中。接下来,程序读取一个单词word和一个整数k。函数brotherWord将在strVect中查找与word是兄弟单词的单词,并将它们存储在一个名为rtnVect的字符串向量中。如果rtnVect中的单词数量大于1,则输出第k个单词。
整体来说,这段代码实现了一个简单的兄弟单词查找功能。
阅读全文
相关推荐
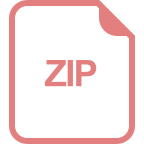
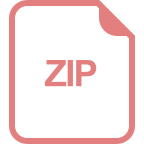
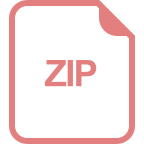













