设计一个测试类testclass,定义生成对象并显示相关运行结果,输出相关信息
时间: 2024-05-05 18:19:18 浏览: 20
好的,您想让我为您设计一个测试类testclass,并定义生成对象并显示相关运行结果的方法,是吗?
是的,您可以按照以下代码来实现:
```
class testclass:
def __init__(self, name):
self.name = name
def show_info(self):
print("This is a test class.")
print("Name of object: {}".format(self.name))
# 生成对象
obj = testclass("TestObject")
# 调用show_info方法并显示相关信息
obj.show_info()
```
以上代码可以生成一个名为TestObject的对象,并显示相应信息。其中,testclass类中的初始化方法__init__用于初始化对象,而show_info方法用于显示相关信息。在使用时,只需要创建一个testclass的对象,然后调用其show_info方法,即可显示相关信息。
请问,您是否满意我的回答?
相关问题
JAVA写一个测试类,定义一个人类的变量a, 生成一个人的对象放入到a变量中去怎么写
您可以按照以下方式编写一个测试类,定义一个人类的变量a,并生成一个人的对象赋值给a变量:
```java
public class Test {
public static void main(String[] args) {
// 定义一个人类的变量a
Person a;
// 生成一个人的对象,并赋值给a变量
a = new Person();
// 其他操作...
}
}
```
在上述代码中,我们首先定义了一个名为`Test`的测试类。在`main`方法中,我们声明了一个人类的变量`a`,并通过`new Person()`创建了一个人的对象,并将其赋值给`a`变量。您可以在生成对象后,根据需要对其进行进一步操作。
请注意,上述代码中的`Person`表示人类,您需要根据您的实际需求编写一个名为`Person`的类。
声明测试类,生成若干个Student类、StudentXW类及StudentBZ类对象,并分别计算它们的评测成绩
假设我们已经有了以下三个类:Student、StudentXW、StudentBZ。其中,Student为基类,StudentXW和StudentBZ为派生类。我们需要在测试类中生成这些对象,并分别计算它们的评测成绩。
首先,我们需要定义Student类,包含学生的基本信息和计算评测成绩的函数calculate_score()。
```python
class Student:
def __init__(self, name, age, score):
self.name = name
self.age = age
self.score = score
def calculate_score(self):
# 计算评测成绩的公式
return self.score * 1.2
```
接下来,我们需要定义StudentXW和StudentBZ类,它们分别继承自Student类。这里我们假设StudentXW和StudentBZ的评测成绩计算方式与Student类不同。
```python
class StudentXW(Student):
def calculate_score(self):
# 计算评测成绩的公式
return self.score * 1.5 + 10
class StudentBZ(Student):
def calculate_score(self):
# 计算评测成绩的公式
return self.score * 1.3 + 20
```
最后,我们需要在测试类中生成若干个对象,并分别计算它们的评测成绩。
```python
class Test:
def __init__(self):
# 生成对象
self.student1 = Student("张三", 18, 90)
self.student2 = StudentXW("李四", 19, 85)
self.student3 = StudentBZ("王五", 20, 95)
def run(self):
# 计算评测成绩
score1 = self.student1.calculate_score()
score2 = self.student2.calculate_score()
score3 = self.student3.calculate_score()
# 输出结果
print(f"{self.student1.name}的评测成绩为{score1}")
print(f"{self.student2.name}的评测成绩为{score2}")
print(f"{self.student3.name}的评测成绩为{score3}")
```
这样,我们就完成了测试类的编写。可以通过以下代码运行程序,查看结果。
```python
test = Test()
test.run()
```
输出结果如下:
```
张三的评测成绩为108.0
李四的评测成绩为132.5
王五的评测成绩为133.0
```
相关推荐
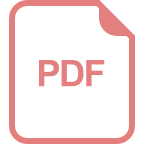
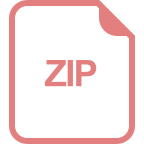












