用C语言单链表编写一个算法 要求可以实现增删改查和联系人分组和置顶联系人功能
时间: 2024-03-09 16:50:48 浏览: 20
好的,下面是一个基于单链表的通讯录管理系统的实现,包括增删改查、联系人分组和置顶联系人功能:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义联系人结构体
typedef struct contact {
char name[20]; // 姓名
char phone[20]; // 电话
char email[30]; // 邮箱
char group[20]; // 分组
int top; // 是否置顶
struct contact *next; // 指向下一个联系人的指针
} Contact;
// 初始化通讯录
Contact *initContact() {
Contact *head = (Contact*)malloc(sizeof(Contact));
strcpy(head->name, "");
strcpy(head->phone, "");
strcpy(head->email, "");
strcpy(head->group, "");
head->top = 0;
head->next = NULL;
return head;
}
// 添加联系人
void addContact(Contact *head) {
Contact *p = (Contact*)malloc(sizeof(Contact));
printf("请输入姓名:");
scanf("%s", p->name);
printf("请输入电话:");
scanf("%s", p->phone);
printf("请输入邮箱:");
scanf("%s", p->email);
printf("请输入分组:");
scanf("%s", p->group);
printf("是否置顶?(1表示是,0表示否)");
scanf("%d", &p->top);
p->next = NULL;
Contact *temp = head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = p;
printf("添加联系人成功!\n\n");
}
// 显示所有联系人
void showContacts(Contact *head) {
Contact *p = head->next;
if (p == NULL) {
printf("通讯录为空!\n\n");
return;
}
printf("姓名\t电话\t邮箱\t分组\t置顶\n");
while (p != NULL) {
printf("%s\t%s\t%s\t%s\t%d\n", p->name, p->phone, p->email, p->group, p->top);
p = p->next;
}
printf("\n");
}
// 查找联系人
void findContact(Contact *head) {
char name[20];
printf("请输入要查找的联系人姓名:");
scanf("%s", name);
Contact *p = head->next;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
printf("姓名\t电话\t邮箱\t分组\t置顶\n");
printf("%s\t%s\t%s\t%s\t%d\n", p->name, p->phone, p->email, p->group, p->top);
printf("\n");
return;
}
p = p->next;
}
printf("通讯录中没有此联系人!\n\n");
}
// 修改联系人
void modifyContact(Contact *head) {
char name[20];
printf("请输入要修改的联系人姓名:");
scanf("%s", name);
Contact *p = head->next;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
printf("请输入电话:");
scanf("%s", p->phone);
printf("请输入邮箱:");
scanf("%s", p->email);
printf("请输入分组:");
scanf("%s", p->group);
printf("是否置顶?(1表示是,0表示否)");
scanf("%d", &p->top);
printf("修改联系人成功!\n\n");
return;
}
p = p->next;
}
printf("通讯录中没有此联系人!\n\n");
}
// 删除联系人
void deleteContact(Contact *head) {
char name[20];
printf("请输入要删除的联系人姓名:");
scanf("%s", name);
Contact *p = head->next;
Contact *prev = head;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
prev->next = p->next;
free(p);
printf("删除联系人成功!\n\n");
return;
}
prev = p;
p = p->next;
}
printf("通讯录中没有此联系人!\n\n");
}
// 分组联系人
void groupContact(Contact *head) {
char group[20];
printf("请输入要分组的联系人姓名:");
scanf("%s", group);
Contact *p = head->next;
while (p != NULL) {
if (strcmp(p->name, group) == 0) {
printf("请输入分组名:");
scanf("%s", p->group);
printf("分组成功!\n\n");
return;
}
p = p->next;
}
printf("通讯录中没有此联系人!\n\n");
}
// 置顶联系人
void topContact(Contact *head) {
char name[20];
printf("请输入要置顶的联系人姓名:");
scanf("%s", name);
Contact *p = head->next;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
p->top = 1;
printf("置顶成功!\n\n");
return;
}
p = p->next;
}
printf("通讯录中没有此联系人!\n\n");
}
// 取消置顶联系人
void untopContact(Contact *head) {
char name[20];
printf("请输入要取消置顶的联系人姓名:");
scanf("%s", name);
Contact *p = head->next;
while (p != NULL) {
if (strcmp(p->name, name) == 0) {
p->top = 0;
printf("取消置顶成功!\n\n");
return;
}
p = p->next;
}
printf("通讯录中没有此联系人!\n\n");
}
int main() {
Contact *head = initContact(); // 初始化通讯录
int choice;
while (1) {
printf("===============通讯录管理系统===============\n");
printf("1.添加联系人\t2.查找联系人\t3.修改联系人\n");
printf("4.删除联系人\t5.显示所有联系人\t6.分组联系人\n");
printf("7.置顶联系人\t8.取消置顶联系人\t9.退出系统\n");
printf("============================================\n");
printf("请选择操作:");
scanf("%d", &choice);
switch (choice) {
case 1:
addContact(head);
break;
case 2:
findContact(head);
break;
case 3:
modifyContact(head);
break;
case 4:
deleteContact(head);
break;
case 5:
showContacts(head);
break;
case 6:
groupContact(head);
break;
case 7:
topContact(head);
break;
case 8:
untopContact(head);
break;
case 9:
printf("感谢使用通讯录管理系统,再见!\n");
exit(0);
default:
printf("输入有误,请重新输入!\n\n");
break;
}
}
return 0;
}
```
在这个实现中,通讯录使用单链表进行存储,每个联系人使用一个结构体来表示,并且结构体中包含了姓名、电话、邮箱、分组和是否置顶等属性。在初始化通讯录时,我们使用一个头节点来表示整个单链表,并将其初始化为空链表。在实现各种功能时,我们需要遍历单链表来找到对应的联系人或位置,然后进行操作。
相关推荐
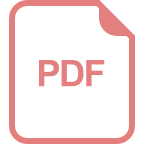














