单链表的增删改查如何用c语言实现
时间: 2023-04-09 19:01:11 浏览: 82
对于这个问题,我可以回答。单链表可以用结构体和指针来实现。具体实现方法如下:
1. 增加节点:创建一个新节点,将新节点的指针指向原来的节点,再将原来的节点的指针指向新节点。
2. 删除节点:找到要删除的节点的前一个节点,将前一个节点的指针指向要删除节点的下一个节点,再释放要删除的节点。
3. 修改节点:找到要修改的节点,将节点的数据域修改为新的值。
4. 查找节点:从头节点开始遍历链表,直到找到要查找的节点为止。
以上就是单链表的增删改查的实现方法。
相关问题
单链表实现增删改查c语言
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
void insertFront(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = (*head_ref);
(*head_ref) = new_node;
}
void insertAfter(struct Node* prev_node, int new_data) {
if (prev_node == NULL) {
printf("Previous node cannot be NULL\n");
return;
}
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
new_node->data = new_data;
new_node->next = prev_node->next;
prev_node->next = new_node;
}
void insertEnd(struct Node** head_ref, int new_data) {
struct Node* new_node = (struct Node*)malloc(sizeof(struct Node));
struct Node* last = (*head_ref);
new_node->data = new_data;
new_node->next = NULL;
if (*head_ref == NULL) {
*head_ref = new_node;
return;
}
while (last->next != NULL)
last = last->next;
last->next = new_node;
return;
}
void deleteNode(struct Node** head_ref, int key) {
struct Node* temp = *head_ref, *prev;
if (temp != NULL && temp->data == key) {
*head_ref = temp->next;
free(temp);
return;
}
while (temp != NULL && temp->data != key) {
prev = temp;
temp = temp->next;
}
if (temp == NULL)
return;
prev->next = temp->next;
free(temp);
}
void modifyNode(struct Node* node, int old_data, int new_data) {
while (node != NULL) {
if (node->data == old_data) {
node->data = new_data;
return;
}
node = node->next;
}
printf("%d not found in the list\n", old_data);
}
int main() {
struct Node* head = NULL;
insertEnd(&head, 1);
insertFront(&head, 2);
insertFront(&head, 3);
insertEnd(&head, 4);
insertAfter(head->next, 5);
printf("Original List: ");
printList(head);
deleteNode(&head, 3);
printf("List after deleting 3: ");
printList(head);
modifyNode(head, 2, 6);
printf("List after modifying 2 to 6: ");
printList(head);
return 0;
}
单链表的增删改查用c语言怎么写
对于这个问题,我可以回答。单链表的增删改查可以用C语言来实现。具体实现方法可以参考以下代码:
//定义单链表节点结构体
typedef struct Node{
int data;
struct Node *next;
}Node;
//创建单链表
Node* createList(){
Node *head = (Node*)malloc(sizeof(Node));
head->next = NULL;
return head;
}
//插入节点
void insertNode(Node *head, int data){
Node *p = (Node*)malloc(sizeof(Node));
p->data = data;
p->next = head->next;
head->next = p;
}
//删除节点
void deleteNode(Node *head, int data){
Node *p = head->next;
Node *pre = head;
while(p != NULL){
if(p->data == data){
pre->next = p->next;
free(p);
break;
}
pre = p;
p = p->next;
}
}
//修改节点
void updateNode(Node *head, int oldData, int newData){
Node *p = head->next;
while(p != NULL){
if(p->data == oldData){
p->data = newData;
break;
}
p = p->next;
}
}
//查找节点
Node* searchNode(Node *head, int data){
Node *p = head->next;
while(p != NULL){
if(p->data == data){
return p;
}
p = p->next;
}
return NULL;
}
//释放链表
void freeList(Node *head){
Node *p = head->next;
while(p != NULL){
Node *temp = p;
p = p->next;
free(temp);
}
free(head);
}
以上就是单链表的增删改查的C语言实现方法。
相关推荐
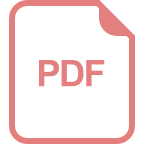












